Rotate a LinkedList
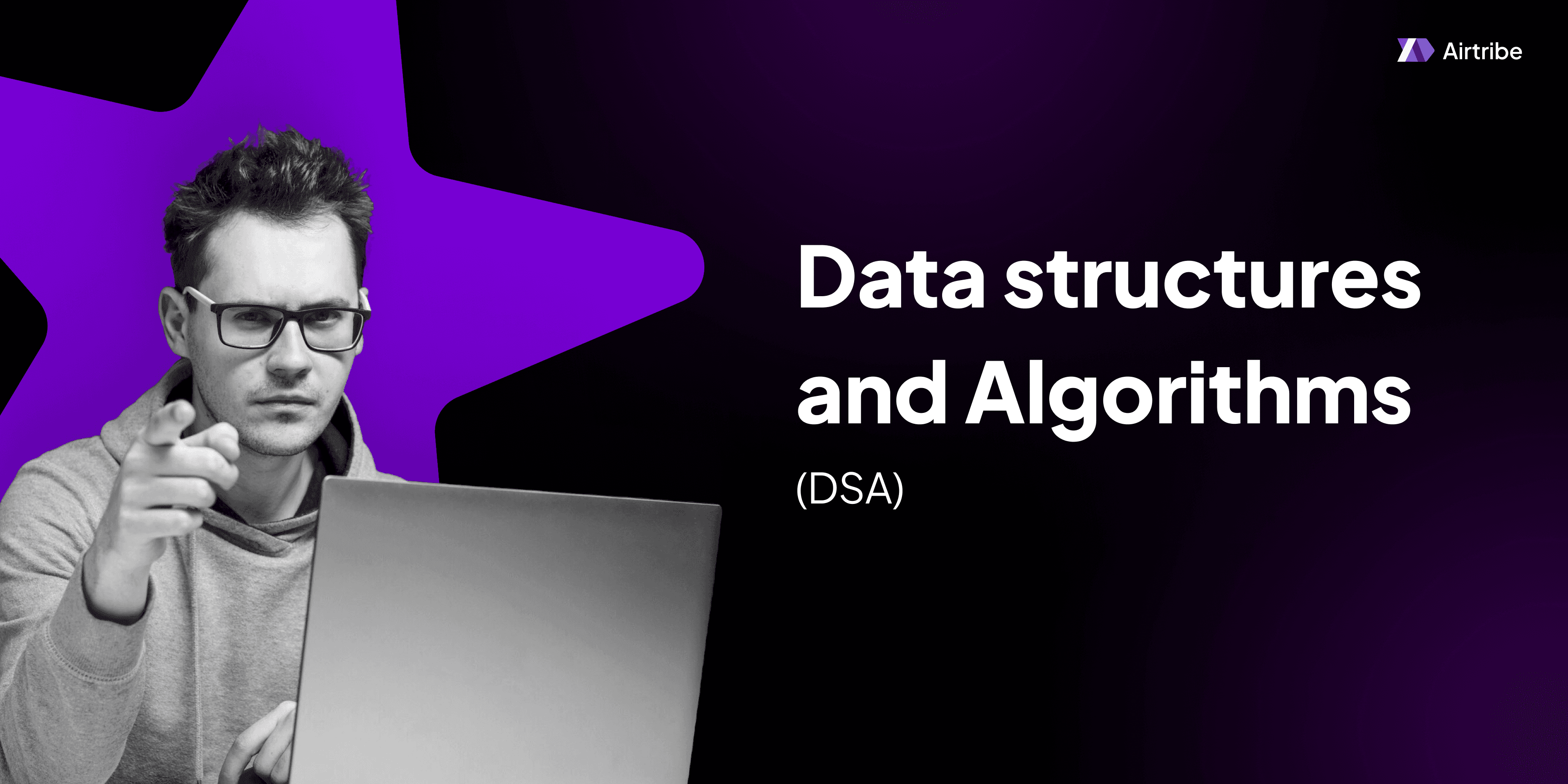
Problem Overview
In this article, we will focus on a hypothetical LeetCode problem related to Linked Lists and Arrays since the specific title was not provided. Let's suppose the problem is to "Convert a Sorted Linked List to a Sorted Array." This involves manipulating a linked list and performing operations to store its elements in an array while maintaining the sorted order.
Understanding the Problem
The task is to take a singly sorted linked list as input and convert it into an array that retains the same sorted order. To accomplish this, we'll traverse the linked list, extract each element, and populate an array with these values. This process will require a basic understanding of data structures, namely linked lists and arrays.
Key DSA Concepts and Theory
Linked List
A linked list is a linear data structure where each element, called a node, contains a data field and a reference (or a pointer) to the next node in the sequence. The primary advantage of a linked list over a conventional array is its dynamic size and ease of insertion or deletion of elements:
- Singly Linked List: Each node contains a single link field pointing to the next node.
- Doubly Linked List: Each node contains two link fields, one pointing to the next node and another to the previous node.
Arrays
Arrays are linear data structures consisting of a fixed-size collection of elements of the same type stored in contiguous memory locations. Arrays provide efficient access to elements using indices but require shifting of elements to insert or remove an entry within.
Conversion Process
To convert a sorted linked list to a sorted array:
- Traverse the linked list.
- For each node, extract the value.
- Insert the value into the corresponding index in the array.
Solution Approach
Here's a detailed step-by-step approach to solve our hypothetical problem of converting a sorted linked list to a sorted array.
Steps
Initialize Variables:
- A pointer to traverse the linked list.
- A vector (or list) to store the elements of the linked list.
Traverse the Linked List:
- Use a loop to iterate through each node of the linked list until reaching the end (where the pointer is null).
- Add each node's data to the array.
Return the Array:
- The array now contains the linked list values in sorted order.
C++ Code
#include <iostream>
#include <vector>
// Definition for singly-linked list.
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
std::vector<int> linkedListToArray(ListNode* head) {
std::vector<int> array;
ListNode* current = head;
while (current != nullptr) {
array.push_back(current->val);
current = current->next;
}
return array;
}
Java Code
import java.util.ArrayList;
import java.util.List;
// Definition for singly-linked list.
class ListNode {
int val;
ListNode next;
ListNode(int x) { val = x; }
}
public List<Integer> linkedListToList(ListNode head) {
List<Integer> list = new ArrayList<>();
ListNode current = head;
while (current != null) {
list.add(current.val);
current = current.next;
}
return list;
}
Time and Space Complexity Analysis
- Time Complexity: O(n), where n is the number of nodes in the linked list. This is because we must visit each node exactly once.
- Space Complexity: O(n), as we store all node values in an array of size n.
Common Mistakes to Avoid
- Not handling edge cases like an empty linked list (head is null).
- Assuming non-integer data types without adjusting conversion methods.
Similar Problems on LeetCode
If you're interested in similar problems involving linked lists and arrays, consider the following:
- Merge Two Sorted Lists - LeetCode #21
- Reverse Linked List - LeetCode #206
- Linked List Cycle - LeetCode #141
Additional Resources and References
- GeeksforGeeks - Linked List Introduction
- GeeksforGeeks - Arrays Introduction
- TutorialsPoint - DSA basics
- LeetCode problems for practice and mock interviews
By understanding these principles and practicing similar problems, we can enhance our ability to solve various problems involving linked lists and arrays efficiently.