Reverse a LinkedList in groups of size k.
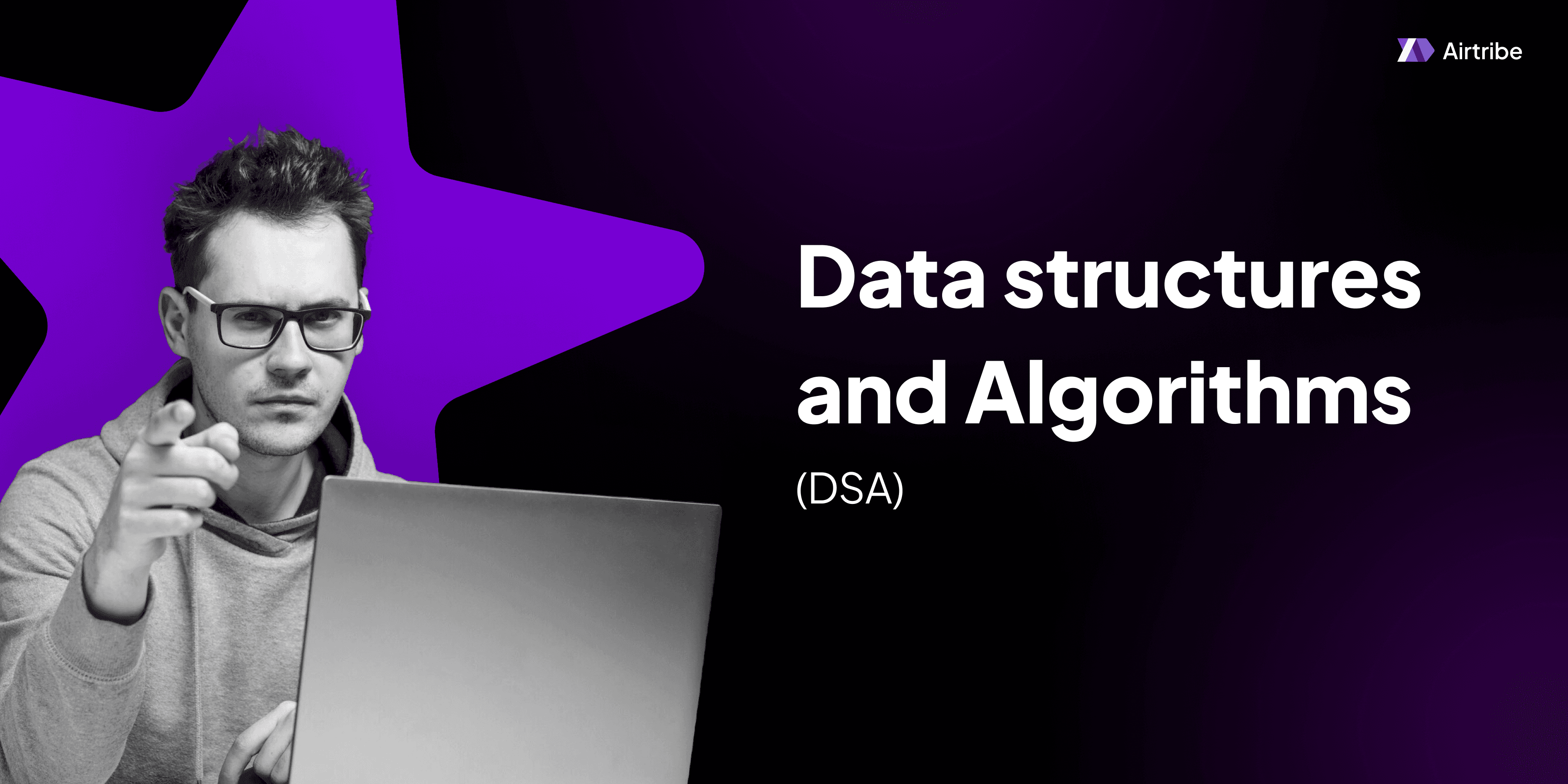
Problem Overview
The problem "Reverse Nodes in k-Group" can be found on LeetCode and involves manipulating a linked list. The goal is to reverse the nodes of a linked list k
at a time and return its modified list. If the number of nodes is not a multiple of k
then the last remaining nodes, in the end, should remain as they are.
Understanding the Problem
Given a linked list, we’re tasked with reversing every contiguous segment of k
nodes. The function should return a modified version of the original linked list where the nodes have been reversed in groups of k
. For instance, given the linked list 1 -> 2 -> 3 -> 4 -> 5
with k = 2
, the new linked list should be 2 -> 1 -> 4 -> 3 -> 5
.
We need to ensure:
- If the number of nodes is not a multiple of
k
, they should be left as is. - We only reverse nodes within full chunks of size
k
.
Key DSA Concepts and Theory
Linked List
A linked list is a linear data structure, in which the elements are stored in nodes, and each node points to the next node via a pointer or reference. It is particularly effective for scenarios where frequent insertion and deletion operations are needed, as these operations can be performed in constant time.
Reverse a Linked List in Groups
For reversing a segment of nodes in a linked list, understanding how to manipulate pointers to change the direction of the list is crucial. When reversing a linked list section, you essentially alter the pointers so that they point in the opposite direction.
Solution Approach
To address this problem, we iterate through the linked list in segments of k
. For each segment, we reverse the nodes and connect the reversed segment back to the main list. Let's break this down further:
Count Nodes: First, ascertain the total number of nodes. If
k
surpasses the number of nodes, leave the list as is.Segment Reversal: For each full segment of size
k
, reverse the segment using three pointers:prev
,curr
, andnext
.Link Segments: After reversing a segment, reconnect it to the previous and the next parts of the list to maintain list continuity.
Here's a detailed implementation in both C++ and Java:
C++ Code Implementation
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
if (!head || k == 1) return head;
ListNode* dummy = new ListNode(0);
dummy->next = head;
ListNode *curr = head, *prev = dummy, *next = nullptr;
int count = 0;
while (curr) {
curr = curr->next;
count++;
}
while (count >= k) {
curr = prev->next;
next = curr->next;
for (int i = 1; i < k; ++i) {
curr->next = next->next;
next->next = prev->next;
prev->next = next;
next = curr->next;
}
prev = curr;
count -= k;
}
return dummy->next;
}
};
Java Code Implementation
class Solution {
public ListNode reverseKGroup(ListNode head, int k) {
if (head == null || k == 1) return head;
ListNode dummy = new ListNode(0);
dummy.next = head;
ListNode curr = head, prev = dummy, next = null;
int count = 0;
while (curr != null) {
curr = curr.next;
count++;
}
while (count >= k) {
curr = prev.next;
next = curr.next;
for (int i = 1; i < k; i++) {
curr.next = next.next;
next.next = prev.next;
prev.next = next;
next = curr.next;
}
prev = curr;
count -= k;
}
return dummy.next;
}
}
Time and Space Complexity Analysis
Time Complexity
The time complexity for both implementations is (O(n)), where (n) is the number of nodes in the linked list. This results from looping over the list to count nodes and to reverse each k-length segment.
Space Complexity
The space complexity is (O(1)) in both C++ and Java implementations. This is because the reversal process utilizes only a fixed amount of extra space (pointers), and no additional data structures are used.
Common Mistakes to Avoid
- Incorrect Pointer Manipulation: Mismanaging the pointers' directions during segment reversal can lead to loops or disconnects in the list.
- Boundary Conditions: Failing to handle cases where the remaining nodes are less than
k
can lead to unintended behavior or runtime errors. - Not Using a Dummy Node: A dummy node simplifies edge cases, especially when reversing the first segment and makes list continuity easier to manage.
Similar Problems on LeetCode
Additional Resources and References
- "Data Structures and Algorithm Analysis in C++" by Mark Allen Weiss - For foundational concepts in linked lists.
- LeetCode Discuss Forum: Explore community solutions and discussions about this and similar problems.
- GeeksforGeeks: Articles on linked list reversal techniques and algorithms.
This comprehensive guide to solving the "Reverse Nodes in k-Group" problem should help clarify the approach and the concepts required to tackle similar problems. Practicing such problems can significantly enhance your understanding of linked list manipulations and pointer management in programming.