Channel allocations problem
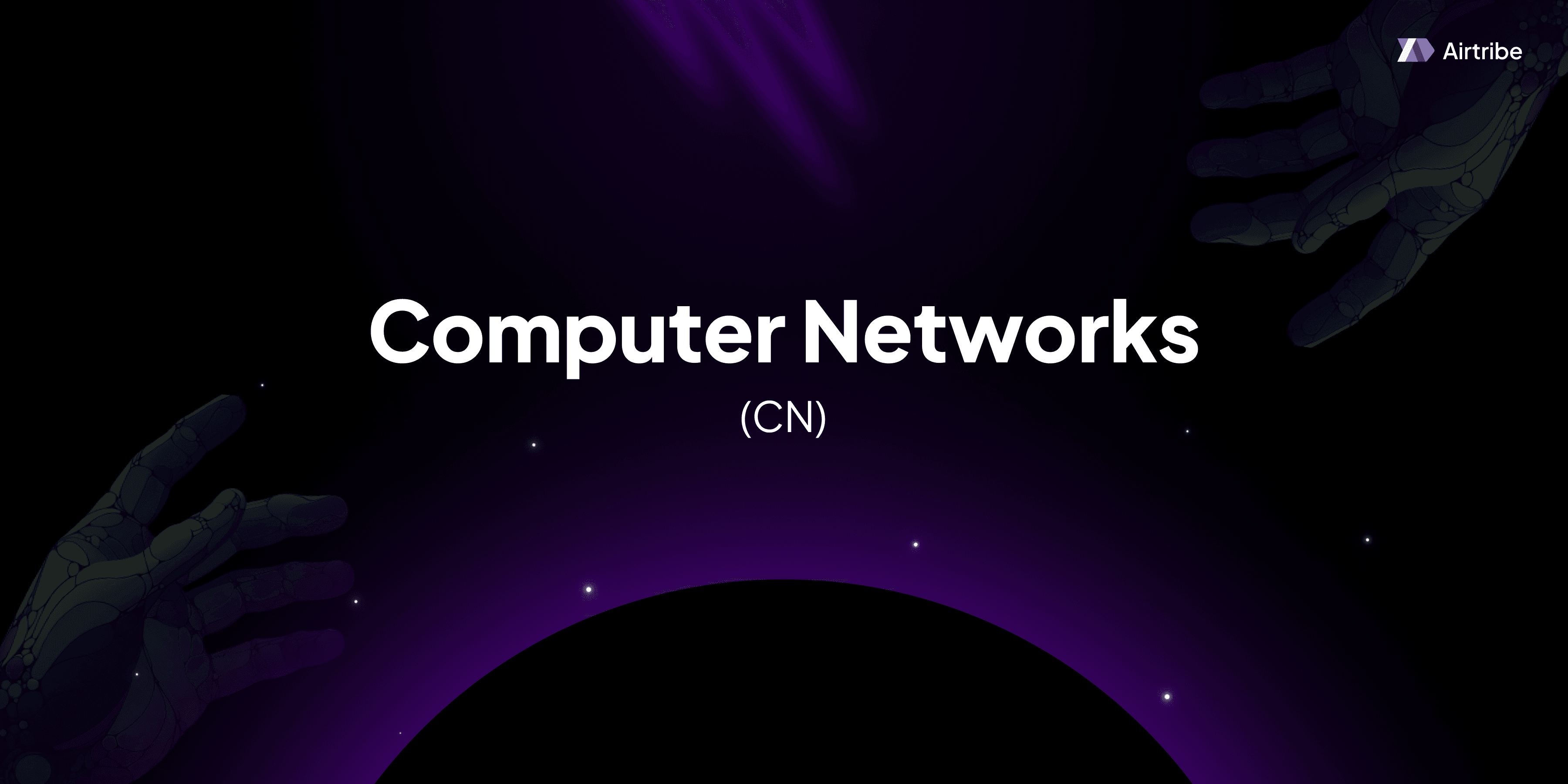
Understanding the Channel Allocation Problem
The channel allocation problem is an essential concern within the data link layer of computer networks. It addresses the challenge of efficiently and effectively distributing communication channels among various users or devices, ensuring optimal performance and minimizing interference. As the demand for data transmission increases, managing the limited available bandwidth becomes critical. This article will explore the core concepts, theoretical foundations, and practical solutions to the channel allocation problem.
Core Concepts and Theory
In computer networks, the data link layer is responsible for node-to-node data transfer. Within this layer, the channel allocation problem focuses on distributing the available bandwidth among competing users or devices. This problem is vital because network resources are finite and shared, leading to potential contention when multiple nodes attempt to communicate simultaneously.
Types of Channel Allocation
Static Allocation: Each channel is permanently assigned to a specific user or device. A key benefit of static allocation is predictability; however, it can lead to inefficiencies, particularly when channel usage is low.
Dynamic Allocation: Channels are assigned on a temporary basis based on demand. This method enhances flexibility and efficiency, as it adapts to changing network conditions. Dynamic allocation often employs various algorithms to ensure fair and effective distribution of channels.
Allocation Strategies
Frequency Division Multiple Access (FDMA): The available bandwidth is divided into frequency bands assigned to different users. Each user has exclusive access to a specific frequency range, reducing interference.
Time Division Multiple Access (TDMA): Time is divided into slots, and each user is assigned specific time slots to transmit data. This ensures that users access the channel sequentially, minimizing collisions.
Code Division Multiple Access (CDMA): Users transmit data simultaneously over the entire frequency spectrum, differentiated by unique codes. CDMA increases channel utilization and supports multiple users sharing the same physical channel.
Key Challenges
The primary challenges in channel allocation include:
Channel Congestion: Occurs when multiple users attempt to access the same channel simultaneously, leading to collisions and reduced network efficiency.
Fairness: Ensuring that all users have equitable access to network resources without any singular user monopolizing the bandwidth.
Scalability: As networks grow, allocation mechanisms must effectively handle an increasing number of devices and users.
Practical Applications
Channel allocation is crucial in various scenarios, such as:
Wireless Networks: Efficient allocation is vital in cellular networks and Wi-Fi, where spectrum scarcity requires maximizing channel usage.
Satellite Communications: Given the limited frequencies available, proper channel management ensures optimal usage for uplink and downlink transmissions.
Emergency Communication Systems: These systems rely on robust channel allocation to maintain stable and reliable communication during critical situations.
Code Implementation and Demonstrations
Implementing channel allocation algorithms, such as TDMA, involves simulating user requests and assigning time slots dynamically. Below is a simple Python example implementing a basic TDMA simulation:
import random
# Initialize available slots
total_slots = 10
users = ['UserA', 'UserB', 'UserC', 'UserD']
assigned_slots = {user: [] for user in users}
def allocate_slots():
for user in users:
# Randomly allocate 1-3 slots for each user
slots_requested = random.randint(1, 3)
available_slots = list(set(range(total_slots)) - set(sum(assigned_slots.values(), [])))
assigned_slots[user] = random.sample(available_slots, min(slots_requested, len(available_slots)))
allocate_slots()
print("TDMA Slot Allocation:")
for user, slots in assigned_slots.items():
print(f"{user}: Slots {slots}")
This script demonstrates how slots can be dynamically assigned to multiple users based on random allocation, mimicking a simplified environment for testing TDMA strategies.
Comparison and Analysis
Allocation Method | Strengths | Weaknesses |
---|---|---|
FDMA | Simple implementation, low latency | Inefficient with fluctuating demand |
TDMA | Suited for bursty data, structured | Time-slot wastage, limited users |
CDMA | High capacity, flexible usage | Complex to implement, high power requirements |
Effective channel allocation combines different strategies and adapts them to suit specific network needs based on available resources, user demands, and infrastructure capabilities.
Additional Resources and References
To further explore the channel allocation problem and its solutions, consider the following resources:
- "Data and Computer Communications" by William Stallings: This book provides in-depth insights into various network communication protocols and channel management techniques.
- IEEE Communications Society: Offers a wealth of articles and papers on emerging trends in channel allocation and management strategies.
- "Computer Networking: A Top-Down Approach" by James Kurose and Keith Ross: An excellent resource for understanding networking fundamentals and implementation details.
Understanding and effectively tackling the channel allocation problem is essential for ensuring optimized and uninterrupted data transfer in modern and future communication networks.