Different Layers of the TCP/IP Model
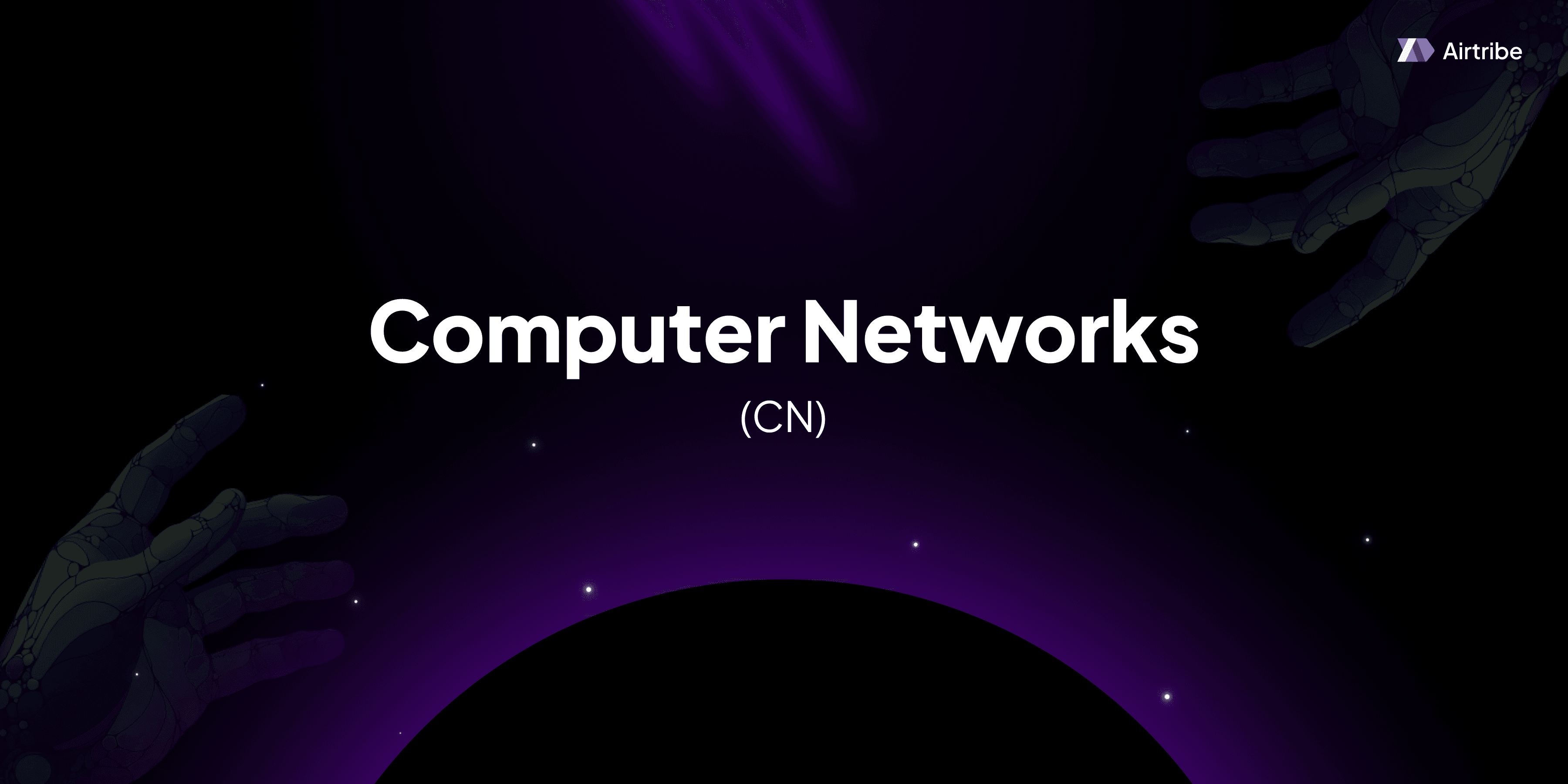
Understanding the Layers of the TCP/IP Model
The TCP/IP model, a cornerstone of computer networks, stands as a robust framework facilitating communication across interconnected devices. This model, an adaptation of the OSI (Open Systems Interconnection) model, structures networking processes into four distinct layers, enabling seamless data transfer over diverse networks. This article delves into the intricacies of these layers, unraveling core concepts, practical applications, and how they compare to the OSI model.
Core Concepts and Theory
The TCP/IP model, also known as the Internet protocol suite, streamlines network communication by abstracting processes into four layers: the Link Layer, Internet Layer, Transport Layer, and Application Layer. Each layer is tasked with specific functions, contributing to the model's efficiency and adaptability.
1. Link Layer
The Link Layer, also known as the Network Interface Layer, is the foundational layer responsible for physical transmission of data packets over network hardware. It encompasses protocols like Ethernet and Wi-Fi that manage data exchanges within the same network or physical link.
- Functions:
- Converts packets into frames for transmission.
- Controls hardware addressing.
- Manages error detection and correction.
2. Internet Layer
The Internet Layer is crucial for routing data packets across different networks. It employs the Internet Protocol (IP) to deliver packets from the source host to the destination host, regardless of the physical network structure.
- Functions:
- Logical addressing with IP addresses.
- Path determination and routing.
- Fragmentation and reassembly of packets.
3. Transport Layer
The Transport Layer ensures reliable communication sessions between devices, multiplexing, and maintains the integrity of transferred data through protocols like Transmission Control Protocol (TCP) and User Datagram Protocol (UDP).
- Functions:
- Segmentation and reassembly of data.
- Flow control and error recovery.
- Connection-oriented (TCP) and connectionless (UDP) communication.
4. Application Layer
At the top of the model, the Application Layer interfaces directly with end-user services and applications. It facilitates various network services by providing protocols for data exchange.
- Functions:
- Supports network applications including HTTP, FTP, SMTP.
- Establishes protocols for user-interface communication.
- Provides data encoding and dialogue implementation.
Practical Applications
The TCP/IP model is integral to daily internet usage, underpinning web browsing, email services, file transfers, and online communication. Understanding these layers enhances network troubleshooting, optimizes protocol configurations, and aids in the design of robust network infrastructures.
Code Implementation and Demonstrations
To practically apply the understanding of the Transport Layer, consider implementing a basic TCP client-server model in Python:
# Simple TCP client-server example
# Server Code
import socket
def start_server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8080))
server_socket.listen(1)
print("Server started and listening...")
connection, address = server_socket.accept()
print(f"Connected to {address}")
while True:
data = connection.recv(1024).decode()
if not data:
break
print(f"Received from client: {data}")
connection.sendall(data.encode())
connection.close()
server_socket.close()
# Client Code
def start_client():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 8080))
message = "Hello, Server!"
client_socket.sendall(message.encode())
data = client_socket.recv(1024).decode()
print(f"Received from server: {data}")
client_socket.close()
These codes demonstrate the principles of connection-oriented data transfer using TCP, showcasing the Transport Layer's effectiveness in ensuring reliable communication.
Comparison and Analysis
While both the TCP/IP and OSI models aim to standardize network communication, the TCP/IP model is more streamlined, reducing the complexity by consolidating functions into four layers. The OSI model, in contrast, comprises seven layers, offering a more granular approach that, while comprehensive, isn't widely implemented.
Feature | TCP/IP Model | OSI Model |
---|---|---|
Layers | 4 | 7 |
Implementation | Widely adopted | Comparative educational |
Flexibility | Practical and simplified | Detailed and theoretical |
Though the OSI model provides detailed guidance applicable in understanding diverse networking architectures, the TCP/IP model remains the workhorse of the Internet due to its straightforward and adaptive design.
Additional Resources and References
To deepen your understanding of the TCP/IP model, consider exploring these resources:
Books:
- "Computer Networks" by Andrew S. Tanenbaum
- "TCP/IP Illustrated" by W. Richard Stevens
Online Courses:
- "Introduction to Networking" on Coursera
- "Networking Basics" by Cisco Networking Academy
Websites such as the Internet Engineering Task Force (IETF) provide extensive documentation on protocols and networking standards that align with the TCP/IP model.
By grasping the principles of the TCP/IP model, Software Development Engineers can design, troubleshoot, and optimize networked systems with efficacy and insight.