Hash Functions
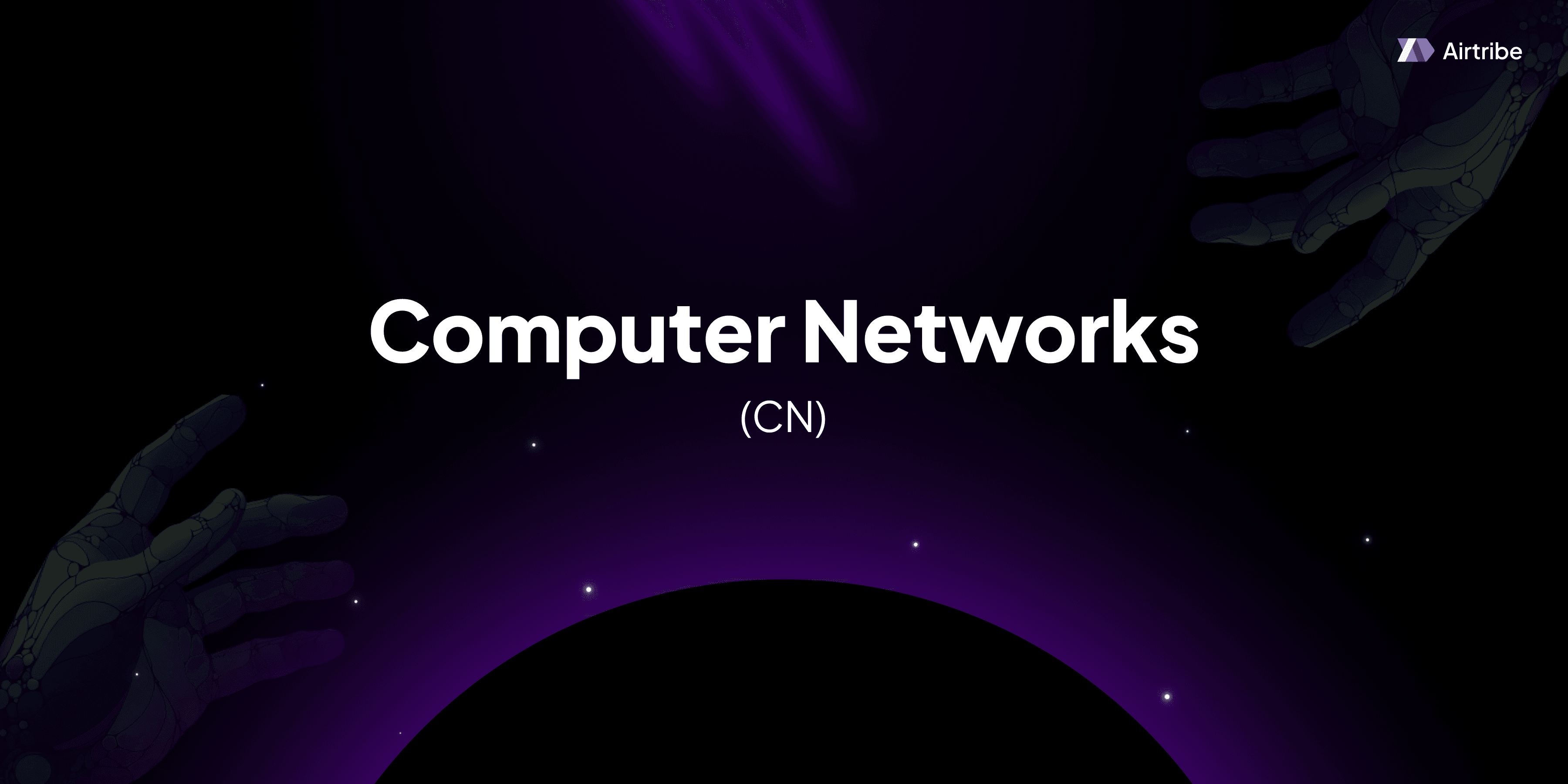
Introduction to Hash Functions
Hash functions are fundamental building blocks in the realm of network security and computer networks. They play a crucial role in ensuring data integrity, authentication, and efficient data retrieval processes. This article delves into the core concepts of hash functions, illustrating their significance in network security and exploring their various applications and implementations.
Core Concepts and Theory
What is a Hash Function?
A hash function is a mathematical algorithm that converts an input (or 'message') into a fixed-size string of bytes. This output, known as the hash value or hash code, is typically a shorter representation of the original input. Hash functions are deterministic, meaning that the same input will always yield the same hash value.
Properties of Hash Functions
- Deterministic: The same input will always produce the same output.
- Fast Computation: The hash value should be calculated quickly.
- Pre-image Resistance: Given a hash value, it should be computationally infeasible to retrieve the original input.
- Small Changes in Input Produce Different Hashes: A slight change in the input should radically change the hash value, a property known as the avalanche effect.
- Collision Resistance: It should be difficult to find two different inputs that produce the same hash value.
Hash Functions in Network Security
Hash functions are pivotal in maintaining data security across networks. They are employed in a variety of security protocols and structures, such as:
- Data Integrity: Hash codes can verify that data hasn't been altered during transmission. By comparing hash values before and after transit, integrity can be confirmed.
- Authentication: Hash functions aid in authenticating users and messages without exposing sensitive data (e.g., passwords).
- Digital Signatures: They are used in the creation and verification of digital signatures, providing non-repudiation and authenticity.
Practical Applications
Hash functions have broad applications in network security, including but not limited to:
- Cryptographic Applications: Used in generating keys and ensuring secure communications.
- Storing Passwords: Securely hashed passwords are stored in databases to prevent unauthorized access.
- Checksums and Hashes for File Verification: Ensure that files and data haven't been tampered with or corrupted.
- Blockchain: Integral to operations like maintaining a decentralized ledger.
Code Implementation and Demonstrations
Example in Python
Below is a simple demonstration of how hash functions can be implemented in Python using the hashlib
library:
import hashlib
# Function to hash a string using SHA-256
def hash_string(input_string):
sha_signature = hashlib.sha256(input_string.encode()).hexdigest()
return sha_signature
# Example usage
original_string = "Hello, Network Security!"
hashed_value = hash_string(original_string)
print(f"Original String: {original_string}")
print(f"Hashed Value: {hashed_value}")
Explanation
This example utilizes the SHA-256 algorithm, a widely-used cryptographic hash function. The hash_string
function takes a string input and returns its hash value. The hexdigest()
method converts the hash into a hexadecimal format, making it suitable for displaying or storing.
Comparison and Analysis
Comparing Hash Algorithms
Factor | MD5 | SHA-1 | SHA-256 |
---|---|---|---|
Length (bits) | 128 | 160 | 256 |
Speed | Fast | Moderate | Slower |
Security | Vulnerable | Broken | Secure |
- MD5: Known for its speed but susceptible to collision attacks.
- SHA-1: Provides longer hashes and increased complexity but has known vulnerabilities.
- SHA-256: Considered secure and widely used for most cryptographic applications.
Choosing the right hash function depends on the balance between security needs and performance constraints.
Additional Resources and References
For further exploration of hash functions and their applications in network security, consider these resources:
Books:
- "Cryptography and Network Security" by William Stallings
- "Network Security Essentials" by William Stallings
Online Articles:
- Cryptographic Hash Functions - A detailed Wikipedia entry.
- NIST’s Hash Function Projects - National Institute of Standards and Technology.
Understanding hash functions and their implementation can significantly enhance your ability to secure networks and create more robust software systems. By mastering these concepts, professionals in the field can develop secure, efficient, and reliable network solutions.