Internet transport layer protocol - TCP
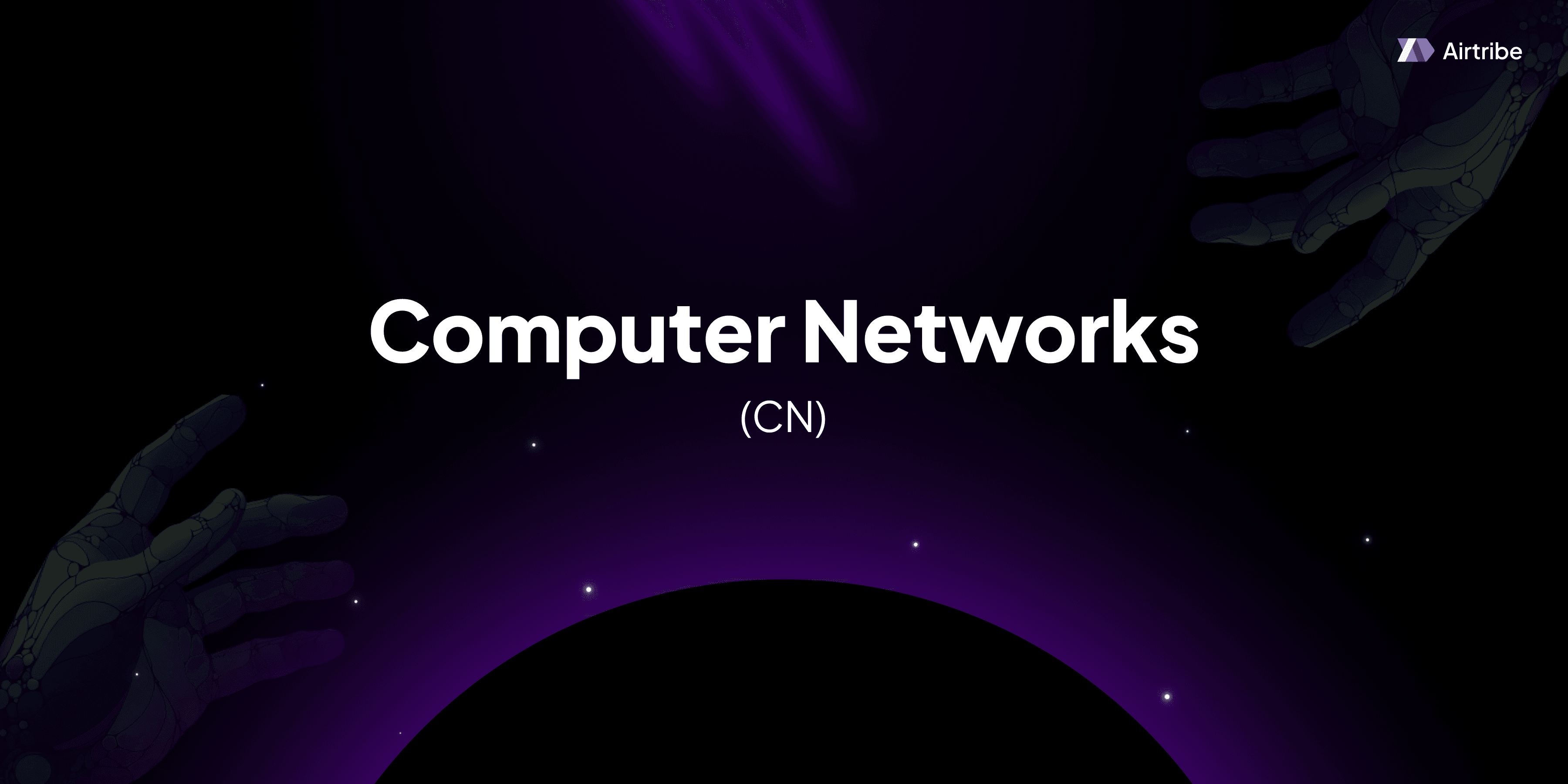
Examining the Internet Transport Layer Protocol: TCP
In the realm of computer networks, the Transport Layer stands crucial for effectively delivering data across diverse systems. Among its key protocols is the Transmission Control Protocol (TCP). Designed for reliability and robust communication, TCP underpins many of the internet's core functionalities, enabling everything from web browsing to secure financial transactions. This article delves into TCP's architecture, operations, and importance in networking.
Core Concepts of TCP
Overview of the Transport Layer
The Transport Layer is the fourth layer in the OSI model and is responsible for end-to-end communication, ensuring complete data transfer from a source to a destination. It manages packetization of data, transfer, and error checking. This layer also facilitates flow control, data integrity, and error recovery, fundamentally crucial for reliable connections.
What is TCP?
TCP, or Transmission Control Protocol, is a staple protocol within this layer. It provides connection-oriented communication, ensuring that data packets are delivered in sequence and without errors. TCP achieves this through mechanisms such as error detection, retransmission, and acknowledgment.
Key Features of TCP
- Connection-Oriented: TCP establishes a connection between sender and receiver before data transfer begins.
- Reliable Data Transfer: It checks data integrity, ensures data segments are not lost or corrupted, and allows for retransmission when errors occur.
- Flow Control: TCP adjusts the rate of data transmission based on the receiver’s capacity, using a windowing mechanism.
- Congestion Control: Prevents network congestion by adjusting data flow to avoid overwhelming the network.
- Orderly Delivery: Guarantees that packets are reassembled in the order they were sent, which is essential for applications requiring strict sequencing.
Theoretical Framework
TCP Segment Structure
TCP data transmission happens via segments. Understanding the TCP segment's structure is critical for grasping its operations:
- Source Port and Destination Port: Identify sending and receiving applications.
- Sequence Number: Ensures packets are delivered in order.
- Acknowledgment Number: Used in acknowledging received segments.
- Flags: Control bits (e.g., ACK, SYN, FIN) manage connection setup and teardown.
- Window Size: Controls flow, indicating the amount of data that the receiver is willing to accept.
- Checksum: Ensures data integrity.
TCP Operations
- Three-Way Handshake: Used to establish a connection (SYN, SYN-ACK, ACK).
- Data Transmission: Segments are sent, acknowledged, and retransmissions occur if necessary.
- Connection Teardown: Gracefully terminates a connection using a four-step handshake (FIN, ACK).
Practical Applications and Code Demonstrations
TCP is pivotal in applications requiring reliable communication, such as:
- Web Browsing: HTTP/HTTPS relies on TCP for sending data between clients and servers.
- Email Transfer: SMTP uses TCP to ensure email data is reliably sent.
- File Transfers: Protocols like FTP depend on TCP for error-free file transmission.
Code Example: Using TCP in Python
Let's see a simple Python example demonstrating a TCP client-server communication.
TCP Server:
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8080))
server_socket.listen(1)
print("Server is listening on port 8080")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address} has been established!")
client_socket.send(bytes("Welcome to the server!", "utf-8"))
client_socket.close()
TCP Client:
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 8080))
message = client_socket.recv(1024)
print(message.decode("utf-8"))
client_socket.close()
This code initiates a simple client-server interaction over TCP, showcasing the connection setup process, data transmission, and connection teardown.
Comparison and Analysis
TCP vs UDP
TCP and UDP (User Datagram Protocol) are both Transport Layer protocols but with distinct differences:
Feature | TCP | UDP |
---|---|---|
Connection Type | Connection-oriented | Connectionless |
Reliability | Reliable, with error checking | Unreliable, no guarantee |
Speed | Slower due to error checking | Faster, with minimal checks |
Use Cases | Web browsers, email, file transfer | Live streaming, online gaming |
Understanding these differences helps in choosing the right protocol based on application requirements.
Additional Resources and References
To deepen your understanding of TCP and its applications, consider the following resources:
- RFC 793: The official specification for TCP.
- "Computer Networking: A Top-Down Approach" by James Kurose: A comprehensive guide on networking concepts.
- Online Networking Courses: Platforms like Coursera and Udemy offer detailed courses on TCP/IP networking.
TCP remains foundational to the internet's architecture, integral for ensuring reliable communication across diverse networked systems. By dissecting TCP's core concepts, operations, and practical applications, this article aims to provide a holistic guide to understanding this pivotal protocol.