Locking protocols
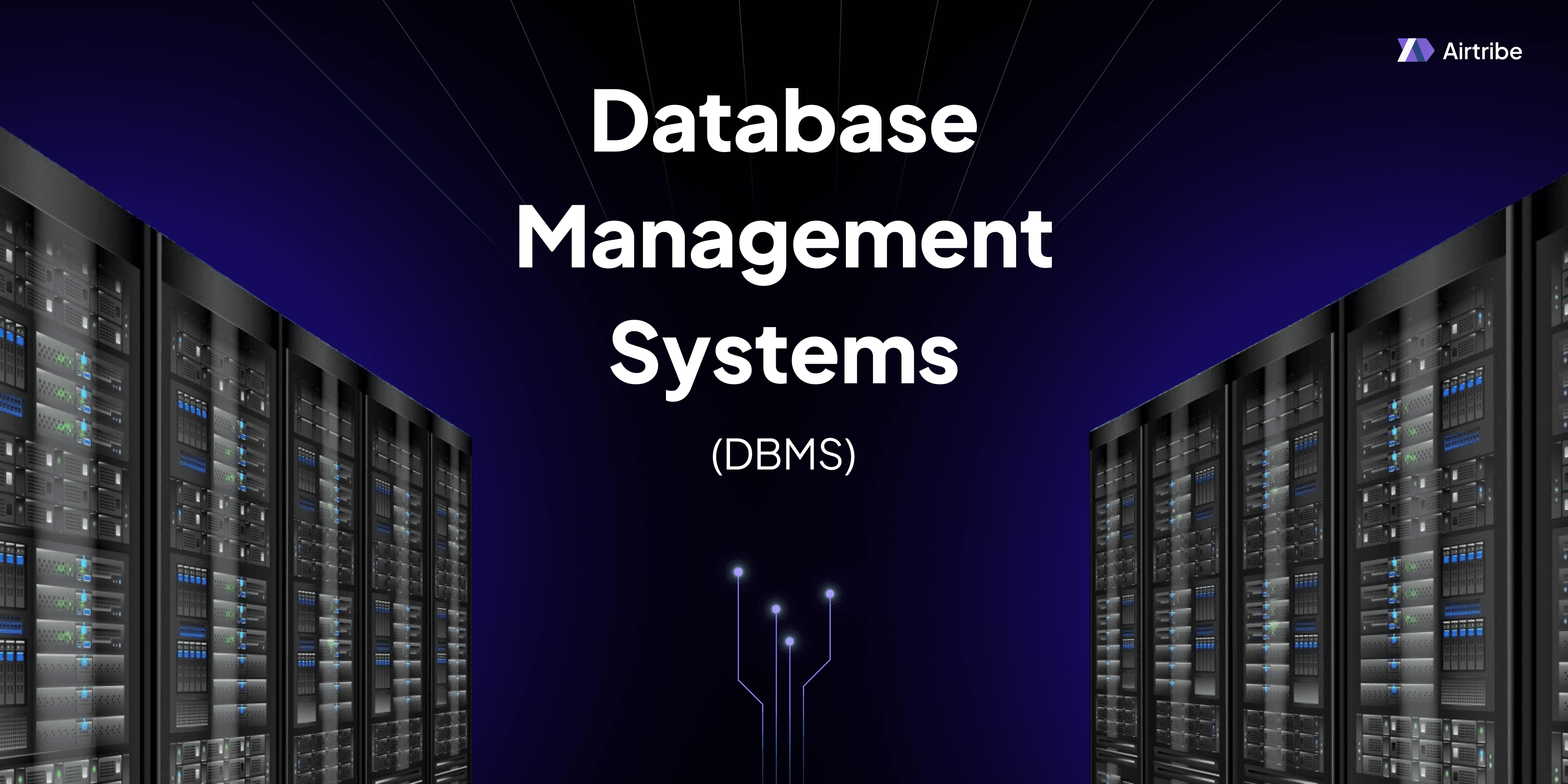
Introduction to Locking Protocols
In the realm of Database Management Systems (DBMS), managing simultaneous transactions and ensuring data consistency is crucial. Locking protocols play a pivotal role in achieving these goals by controlling how concurrent transactions access data. These protocols prevent conflicts arising from concurrent operations, ensuring the integrity of the database.
Core Concepts and Theory
Transactions and Concurrency
Transactions: A transaction is a sequence of operations performed as a single logical unit of work. Transactions possess four key properties commonly referred to as ACID properties: Atomicity, Consistency, Isolation, and Durability.
Concurrency Control: This process ensures that database transactions are executed concurrently without violating data integrity. Without concurrency control, simultaneous transactions might lead to inconsistency, as changes by one transaction can interfere with others.
The Need for Locking
Locking is essential to manage concurrent access to database resources. When a transaction accesses a database item, a lock can be placed on it to control how other transactions access the same item.
Lock Types
Shared Lock (S-Lock): Allows multiple transactions to read a data item but prevents any from modifying it. It's non-exclusive, meaning several transactions can hold a shared lock on the same resource simultaneously.
Exclusive Lock (X-Lock): Allows a single transaction exclusive access to a data item for both reading and modifying. No other transactions can hold any type of lock on the item until the exclusive lock is released.
Locking Protocols
Two-Phase Locking Protocol (2PL): This involves two distinct phases:
- Growing Phase: A transaction may acquire locks but cannot release any locks.
- Shrinking Phase: A transaction can release locks but cannot acquire any new locks.
2PL ensures conflict-serializability, meaning the schedule is equivalent to some serial schedule.
Strict Two-Phase Locking Protocol: A more stringent version of 2PL where a transaction holds all its exclusive locks until it commits. This ensures both conflict-serializability and recoverability.
Rigorous Two-Phase Locking Protocol: Extends strict 2PL by holding all locks (both shared and exclusive) until transaction commit, guaranteeing serializability and cascading aborts prevention.
Practical Applications
Locking protocols are integral in environments where multiple users or applications access the same database simultaneously. They are widely used in:
- Banking Systems: Ensuring consistent balances during fund transfers.
- E-commerce Platforms: Managing inventory levels accurately with concurrent orders.
- Reservation Systems: Preventing double-booking situations by serializing reservation requests.
Code Implementation and Demonstrations
Here is a simple demonstration of how basic locking can work in a pseudo code format:
function transactionProcess(transaction) {
acquireLock(transaction.dataItem, transaction.lockType)
if (transaction.lockType == 'S') {
readData(transaction.dataItem)
} else if (transaction.lockType == 'X') {
writeData(transaction.dataItem)
}
releaseLock(transaction.dataItem)
}
function acquireLock(dataItem, lockType) {
// Logic to check and set the lock on the dataItem
}
function releaseLock(dataItem) {
// Logic to release the lock on the dataItem
}
This is a simplistic approach, but it highlights the fundamental operations involved—acquiring and releasing locks around critical database operations.
Comparison and Analysis
Pros and Cons of Locking Protocols
Protocol | Pros | Cons |
---|---|---|
Two-Phase Locking (2PL) | Ensures serializability | May lead to deadlocks |
Strict 2PL | Prevents cascading rollbacks | Can increase the waiting time for transactions |
Rigorous 2PL | Strong recoverability and avoids cascading aborts | Potentially high resource utilization |
Potential Challenges
Deadlocks: Occur when two or more transactions wait indefinitely for each other to release locks. Strategies like wait-die or wound-wait schemes can mitigate these.
Performance Overhead: Lock management introduces some computational overhead, potentially affecting performance, especially in high-concurrency environments.
Additional Resources and References
To delve deeper into locking protocols, consider exploring the following resources:
- "Database System Concepts" by Abraham Silberschatz, Henry Korth, and Shanmugasundaram Sudarshan
- "Transaction Processing: Concepts and Techniques" by Jim Gray and Andreas Reuter
- Online courses on database management from platforms like Coursera or edX
Understanding locking protocols is essential for database administrators and software developers who aim to build robust, efficient, and consistent database systems. By mastering these concepts, you can ensure that your database transactions are both safe and efficient.