Serializability and concurrency control
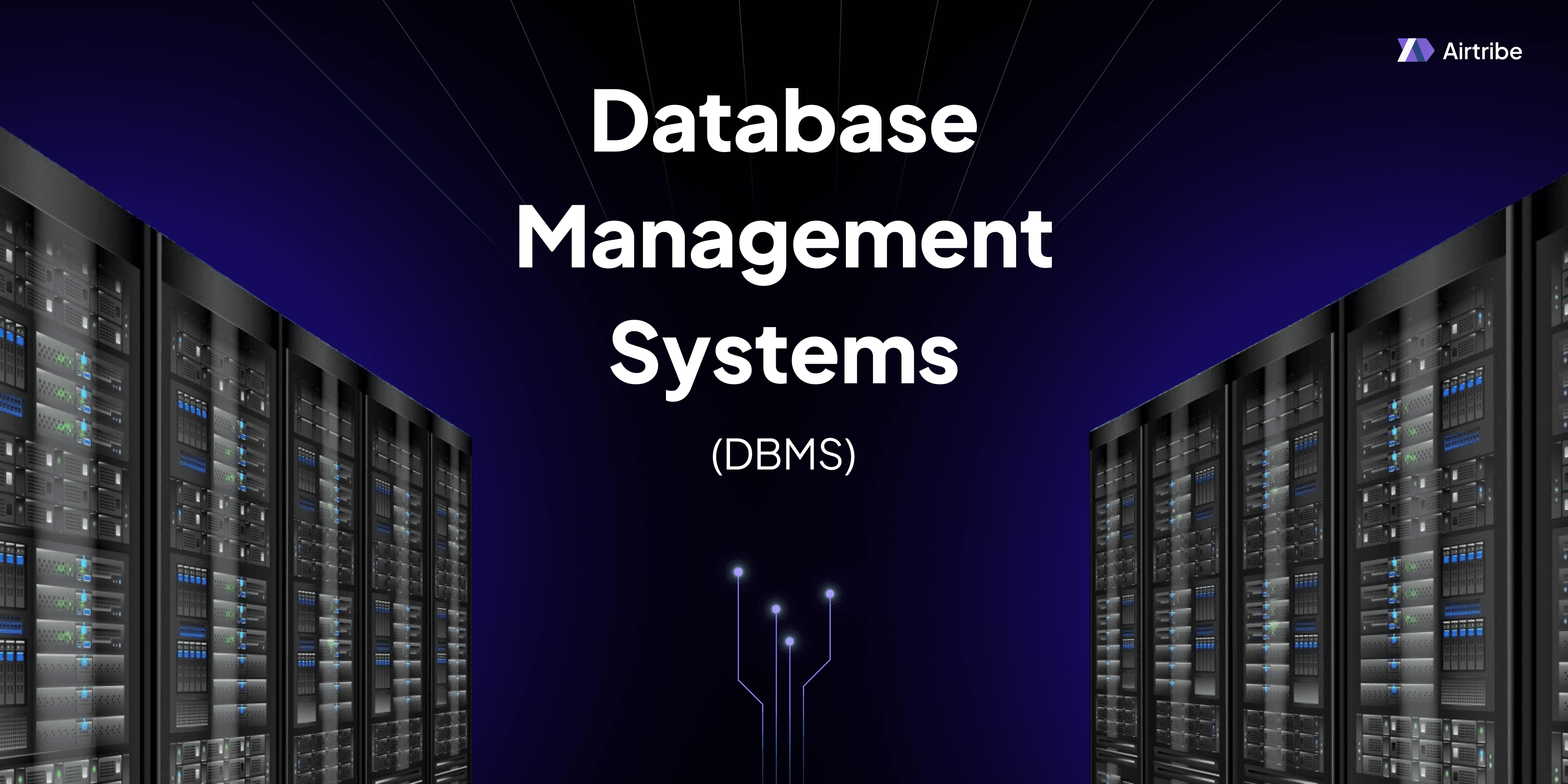
Understanding Serializability and Concurrency Control
In the realm of database management systems (DBMS), ensuring the consistency and integrity of data during concurrent access is paramount. Transactions and concurrency control are two crucial components that play a significant role in managing multiple operations in a database system. This article delves into the concepts of serializability and concurrency control, offering insights into their core principles and real-world applications.
Core Concepts and Theory
Transactions
A transaction in a DBMS is a sequence of operations performed as a single logical unit of work. These operations must adhere to the ACID properties (Atomicity, Consistency, Isolation, Durability) to ensure data reliability:
- Atomicity: Ensures that all operations within a transaction are completed successfully; otherwise, the transaction is aborted.
- Consistency: Guarantees that a transaction transforms the database from one consistent state to another.
- Isolation: Ensures that transactions execute independently without interference, maintaining data legitimacy.
- Durability: Protects completed transactions against system failures.
Concurrency Control
Concurrency control is the process of managing simultaneous transaction execution in a multi-user database environment. It aims to maintain data consistency and integrity while maximizing transaction throughput and system performance. Key challenges in concurrency control include avoiding:
- Lost updates: When two or more transactions update the same data element simultaneously.
- Dirty reads: When a transaction reads data written by a concurrent uncommitted transaction.
- Non-repeatable reads: When a transaction re-reads data it has previously read and finds modifications.
- Phantom reads: When a transaction re-executes a query returning a set of rows satisfying a condition and finds that the set has changed.
Serializability
Serializability is a concurrency control mechanism that ensures transaction schedules are correct and consistent. It is a concept where transactions' interleaved execution results in a database state equivalent to one produced by transactions executed serially, i.e., one after the other. There are two primary types of serializability:
- Conflict Serializability: Ensures that a schedule can be transformed into a serial schedule by swapping non-conflicting operations.
- View Serializability: A more general form allowing more relaxed, albeit less efficient, scheduling compared to conflict serializability.
Lock-Based Protocols
The most common concurrency control mechanism involves lock-based protocols, where transactions must lock data elements before accessing them. Two types of locks are:
- Exclusive Lock (Write Lock): Allows the transaction to read and write a data item, preventing other transactions from accessing it until the lock is released.
- Shared Lock (Read Lock): Allows multiple transactions to read a data item but prevents writing until all shared locks are released.
Locking protocols like Two-Phase Locking (2PL) are used to ensure serializability, where each transaction has a growing phase to acquire all needed locks and a shrinking phase to release them.
Practical Applications
In real-world applications, concurrency control protocols and serializability are crucial in environments where high transaction throughput and data consistency are required. These techniques are widely used in financial systems, online retail, customer relationship management systems, and more.
For instance, in an online booking system, concurrency control ensures multiple users can book tickets simultaneously without any deadlock or inconsistent results due to transaction conflicts.
Code Implementation and Demonstrations
Example: Implementing Locking in SQL
Illustrating transaction management with SQL:
BEGIN TRANSACTION;
-- A shared lock is used for reading
SELECT account_balance
FROM bank_account
WHERE account_id = 123 FOR SHARE;
-- Exclusive lock needed for updating
UPDATE bank_account
SET account_balance = account_balance - 100
WHERE account_id = 123;
COMMIT;
In this example:
- The
SELECT ... FOR SHARE
statement acquires a shared lock, allowing multiple transactions to read simultaneously. - The
UPDATE
statement performs a write operation, requiring an exclusive lock to ensure consistency.
Comparison and Analysis
When comparing lock-based protocols and alternatives like timestamp-based protocols, each has distinct advantages and limitations:
Protocol | Advantages | Limitations |
---|---|---|
Lock-Based | Straightforward implementation, supports both read and write locks | Potential for deadlocks, reduced concurrency |
Timestamp-Based | Avoids deadlocks, provides serializability efficiently | Complexity in implementation, overhead in maintaining timestamps |
These differences influence the protocol choice based on specific application requirements and priorities.
Additional Resources and References
To further explore serializability and concurrency control, consider diving into the following resources:
- "Database System Concepts" by Abraham Silberschatz, Henry Korth, and S. Sudarshan
- "Transactions and Concurrency Control" by ACM Digital Library
- Online Course: Database Management Systems - Coursera by University of Michigan
These materials provide comprehensive insights and advanced knowledge into DBMS functions, offering both theoretical and practical perspectives.
In conclusion, understanding and implementing effective concurrency control mechanisms is crucial for ensuring data consistency and integrity in any database system. Serializability forms the backbone of this process, enabling systems to handle multi-transaction scenarios efficiently and effectively.