SQL clauses
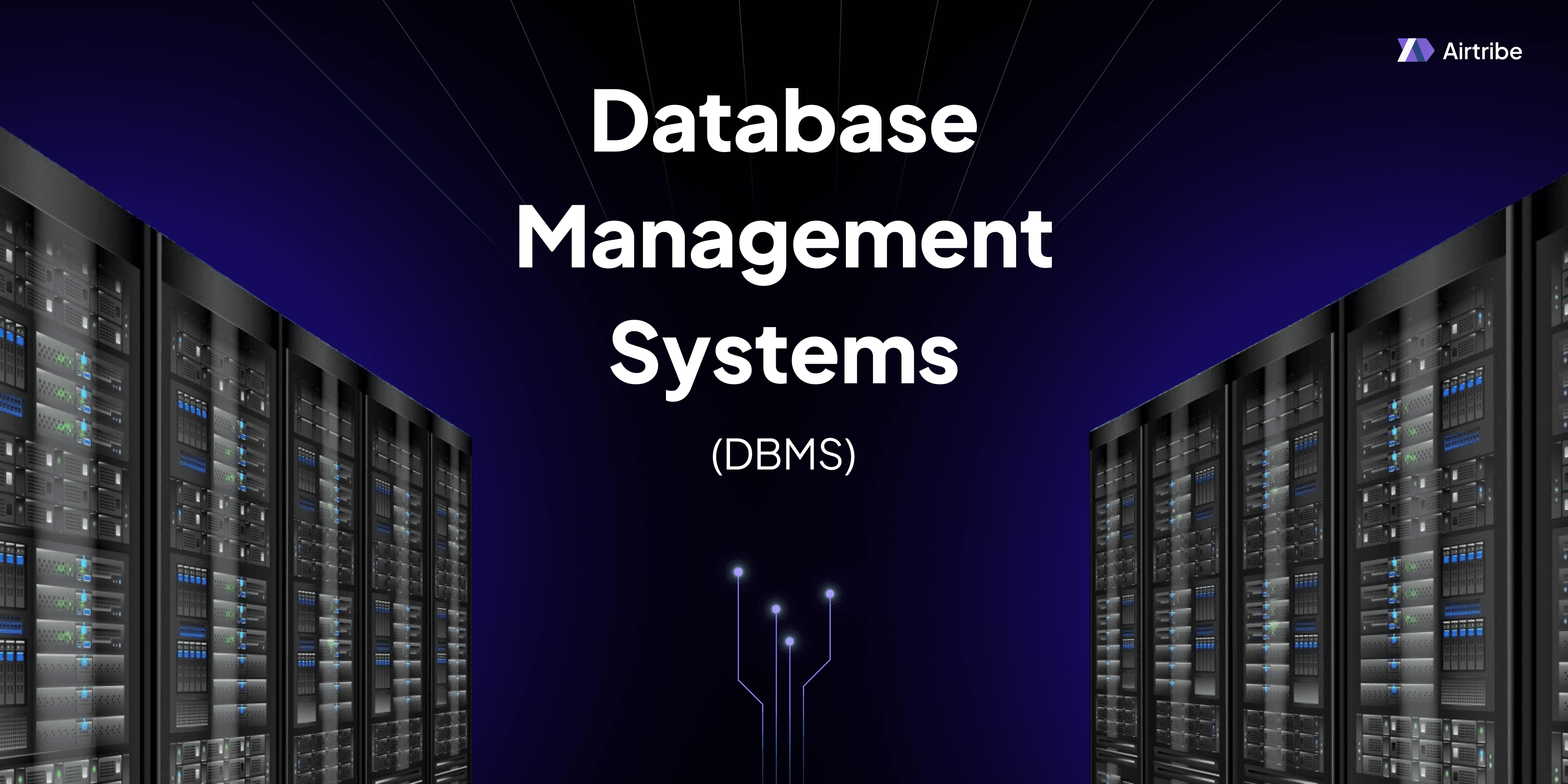
Understanding SQL Clauses
Structured Query Language (SQL) serves as the backbone for interactions with databases, allowing for the retrieval, manipulation, and management of data. At the core of SQL operations are SQL clauses, which help refine and customize queries to meet specific requirements. This article delves into the various SQL clauses, elucidating their roles and applications within database management systems.
Core Concepts and Theory
SQL queries generally consist of various clauses, each serving a unique purpose to form a coherent operation. Understanding these clauses is fundamental for efficient database management and querying.
1. SELECT Clause
The SELECT clause is arguably the most pivotal SQL clause, responsible for specifying the columns to be retrieved from a database table. It forms the foundation for most SQL queries.
Syntax:
SELECT column1, column2, ...
FROM table_name;
2. FROM Clause
The FROM clause indicates the table from which to retrieve or manipulate the data. It is a crucial part of understanding where the data originates in any SQL operation.
Syntax:
SELECT column1, column2, ...
FROM table_name;
3. WHERE Clause
Used to filter records, the WHERE clause conditions the query to return only records that meet certain criteria.
Syntax:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
4. ORDER BY Clause
This clause is employed to sort the result set by one or more columns. The sorting can be done in either ascending (ASC) or descending (DESC) order.
Syntax:
SELECT column1, column2, ...
FROM table_name
ORDER BY column1 DESC, column2 ASC;
5. GROUP BY Clause
GROUP BY organizes rows that have the same values in specified columns into summary rows, like "finding the total revenue for each year".
Syntax:
SELECT column1, aggregate_function(column2)
FROM table_name
GROUP BY column1;
6. HAVING Clause
The HAVING clause was added to SQL because the WHERE keyword cannot be used with aggregate functions. It filters records that work on summarized GROUP BY results.
Syntax:
SELECT column1, aggregate_function(column2)
FROM table_name
GROUP BY column1
HAVING aggregate_function(column2) > value;
7. JOIN Clause
JOIN clause is used to combine rows from two or more tables, based on a related column between them.
Syntax:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column = table2.column;
Practical Applications
SQL clauses are integral in a variety of database operations, including data retrieval, aggregation, and manipulation. By leveraging these clauses, database administrators and developers can execute complex queries that retrieve specific, sorted, and well-organized data sets suitable for analysis and reporting.
Code Implementation and Demonstrations
To illustrate the practical implementation of SQL clauses, consider a simple example using a sample Employees
table:
CREATE TABLE Employees (
ID INT PRIMARY KEY,
Name VARCHAR(100),
Department VARCHAR(100),
Salary INT
);
INSERT INTO Employees VALUES
(1, 'Alice', 'Engineering', 75000),
(2, 'Bob', 'Sales', 55000),
(3, 'Charlie', 'Human Resources', 50000),
(4, 'David', 'Engineering', 80000),
(5, 'Eve', 'Sales', 60000);
Example Query: Find engineering employees earning more than $70,000, sorted by name.
SELECT Name, Salary
FROM Employees
WHERE Department = 'Engineering' AND Salary > 70000
ORDER BY Name ASC;
Additional Resources and References
By comprehending and applying SQL clauses effectively, one can optimize data retrieval processes, enforce business rules, and maintain robust data integrity within any database-driven application. The understanding of these clauses forms the basis for more advanced SQL techniques and design patterns.