Cache
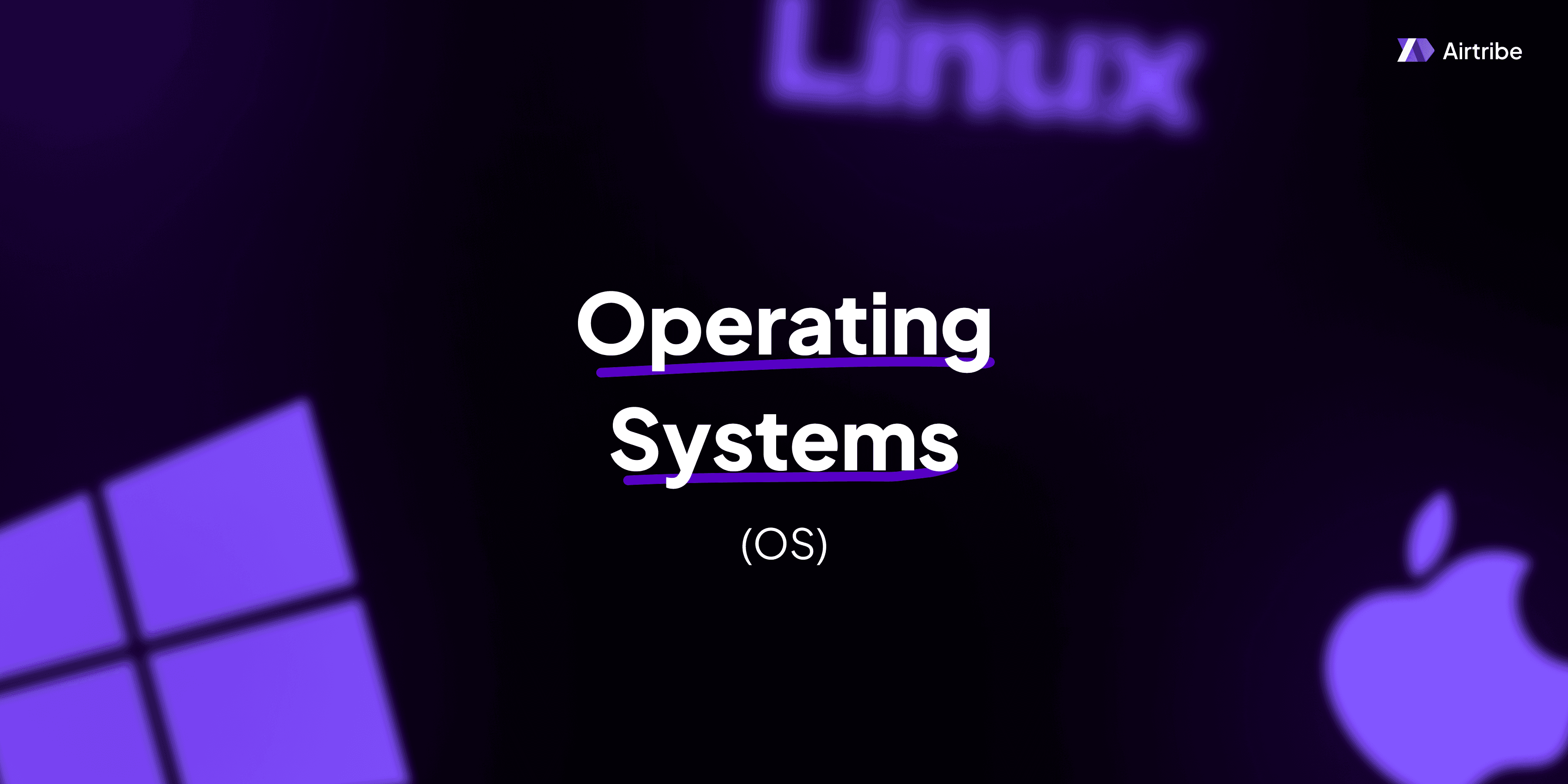
Understanding Cache in Memory Management
Caches play a crucial role in memory management within operating systems and computer architecture, serving as a high-speed data storage layer that stores a subset of data from a larger, slower storage. This article delves into the detailed theory behind cache, its practical applications, implementation details, and a comparison of different caching mechanisms.
Core Concepts and Theory
What is Cache?
A cache is a smaller, faster memory component located between the processor and main memory (RAM), designed to temporarily hold copies of frequently accessed data. By storing these data elements in a cache, systems can access them quickly without needing to reach out to slower main memory, thus improving overall system performance.
How Does Cache Work?
The operation of caching revolves around the principles of locality, including temporal locality and spatial locality. Temporal locality suggests that recently accessed data is likely to be accessed again soon, and spatial locality indicates that data near recently accessed data points is likely to be accessed soon. Caches exploit these principles by storing data elements that have been or are likely to be used soon.
Types of Cache Memory
L1, L2, and L3 Caches: These refer to levels of cache in modern CPUs with L1 being the smallest and fastest located closest to the CPU core, L2 larger and slightly slower, and L3 being the largest and furthest from the core.
Instruction Cache vs. Data Cache: Some caches are designed to store instruction data while others store data related to program execution.
Unified Cache: A cache that handles both instruction and data storage.
Cache Hit and Cache Miss
- Cache Hit: When requested data is found in the cache.
- Cache Miss: When requested data is not found in the cache, requiring retrieval from main memory, following which the cache is updated.
Cache Replacement Policies
Caches have limited space, which makes cache replacement policies crucial to decide which data to evict when the cache is full. Common policies include:
- Least Recently Used (LRU): Evicts the least recently accessed data.
- First In First Out (FIFO): Evicts the oldest data in the cache.
- Least Frequently Used (LFU): Evicts the least frequently accessed data.
Practical Applications
Role in Operating Systems
Caches in operating systems improve the performance of disk storage, network data, and applications by reducing access time and latency. They are fundamental in virtual memory systems to store frequently accessed pages and improve multitasking by reducing thrashing.
Role in Web and Databases
Caches help in storing frequent and recent HTTP responses and database queries, reducing fetch time, and saving network bandwidth and processing power.
Code Implementation and Demonstrations
To demonstrate cache implementation, consider a simple LRU cache using Python's collections module:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key: int) -> int:
if key not in self.cache:
return -1
value = self.cache.pop(key)
self.cache[key] = value
return value
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.pop(key)
elif len(self.cache) >= self.capacity:
self.cache.popitem(last=False)
self.cache[key] = value
# Example usage:
lru_cache = LRUCache(3)
lru_cache.put(1, 1)
lru_cache.put(2, 2)
print(lru_cache.get(1)) # Returns 1
lru_cache.put(3, 3)
lru_cache.put(4, 4)
print(lru_cache.get(2)) # Returns -1 (as 2 was evicted)
Comparison and Analysis
Cache vs. Buffer
- Cache focuses on speeding up data access by temporarily holding copies of data that are recomputed or fetched from a slower source. It leverages temporal and spatial locality for performance.
- Buffer is a temporary storage primarily used to handle data that is being transferred from one place to another, potentially handling differences in transfer speeds.
Cache Performance Metrics
- Hit Ratio: The percentage of cache accesses that result in a cache hit.
- Miss Penalty: The time cost of missing the cache, including the time cost to fetch from slower memory.
Additional Resources and References
Books:
- "Computer Architecture: A Quantitative Approach" by John L. Hennessy and David A. Patterson.
- "Operating System Concepts" by Abraham Silberschatz, Peter B. Galvin, and Greg Gagne.
Online Resources:
Further Reading:
- Analyzing different cache architectures and replacement strategies in modern processors.
- Exploring cache implementations in various programming frameworks such as Redis for web application caching solutions.
Through understanding the cache as a pivotal element in memory management, developers and engineers can optimize software and hardware interactions to boost computational efficiencies significantly.