Contiguous allocation , Paging and Segmentation
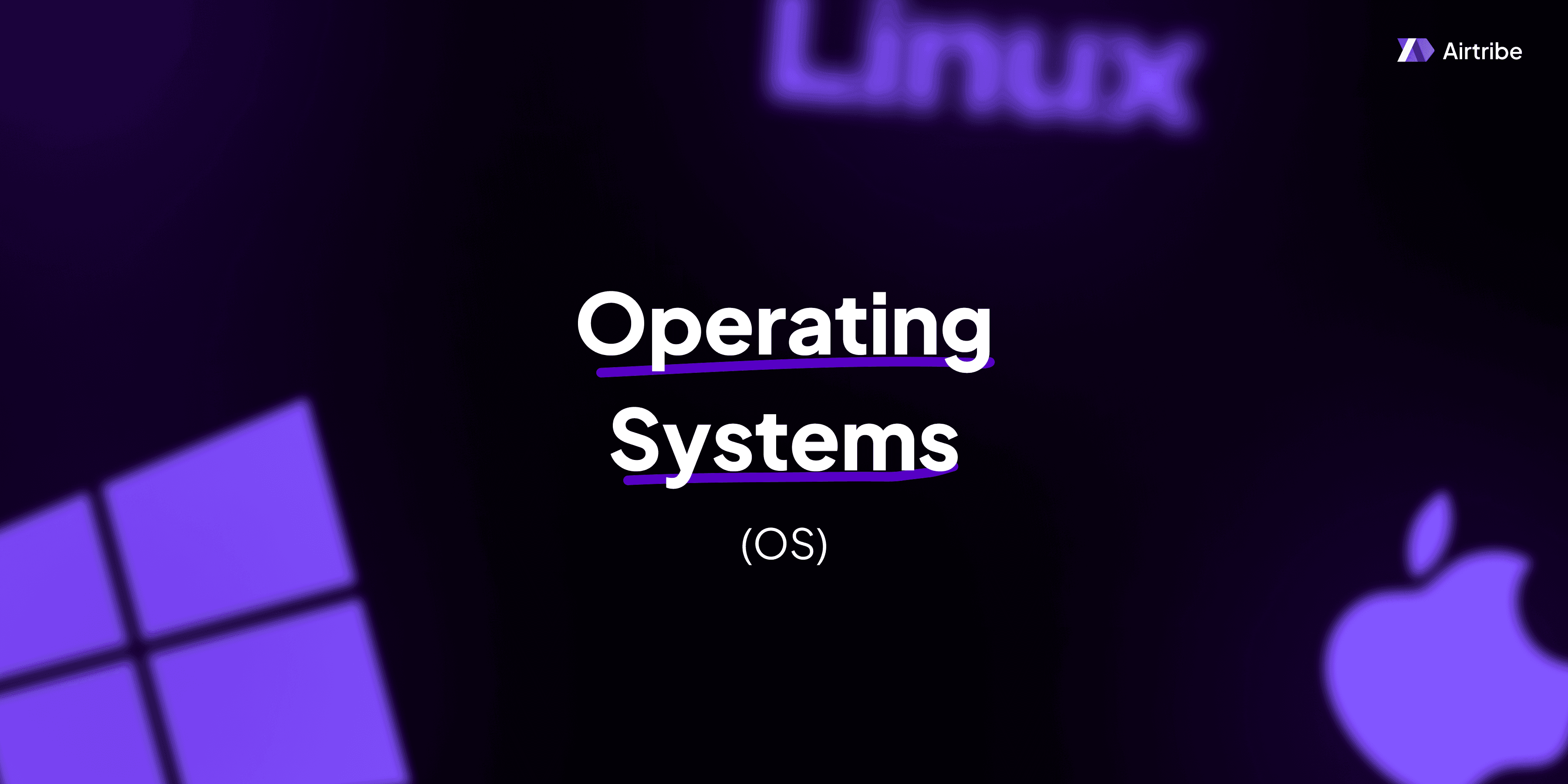
Introduction to Memory Management Techniques
Memory management is a critical aspect of operating systems, responsible for efficiently allocating and managing computer memory. Three primary techniques are utilized for memory management: Contiguous Allocation, Paging, and Segmentation. Each of these methods has its advantages and challenges, influencing system performance. This article delves into each technique, explores their core concepts, and compares them to understand their distinct roles in memory management.
Core Concepts and Theory
Contiguous Allocation
Contiguous allocation involves allocating a single contiguous section of memory to a process. This straightforward method is simple to implement and manage, offering fast access to memory. However, it suffers from fragmentation issues, particularly external fragmentation, where free memory is split into small, non-contiguous blocks, making it difficult to accommodate large processes.
Key Characteristics:
- Simple to implement: Easy to understand and manage.
- Fast access: Direct mapping between logical and physical addresses.
- Fragmentation: Both internal (within an allocated block) and external (unused scattered spaces) fragmentation issues.
Paging
Paging is a non-contiguous memory allocation technique that divides both physical and logical memory into fixed-sized units known as pages and frames, respectively. Each process is divided into pages, which are loaded into any available memory frames.
Key Characteristics:
- No External Fragmentation: Eliminates external fragmentation by using fixed-sized pages.
- Page Table: Maintains a mapping of logical pages to physical frames, adding overhead.
- Internal Fragmentation: May experience internal fragmentation if page size is not well-chosen.
Segmentation
Segmentation divides memory into variable-sized segments based on logical divisions such as functions or data structures. This method supports modular programming by allowing segments to grow independently of fixed boundaries.
Key Characteristics:
- Logical Division: Segments correspond to logical units like functions or arrays.
- Variable Size Segments: Each segment can be different in size, improving memory utilization.
- Segment Table: Maps each segment to a memory location, potentially increasing overhead.
Practical Applications
Each memory allocation technique is suitable for different scenarios:
- Contiguous Allocation: Suitable for simple systems where processes are predictable in size and number, such as embedded systems.
- Paging: Ideal for general-purpose operating systems where demand paging, virtual memory, and protection are crucial.
- Segmentation: Useful in programming environments where logical division of tasks is necessary, and memory access patterns vary significantly.
Code Implementation and Demonstrations
While actual implementation details may vary depending on operating system design and requirements, the following is a high-level overview.
Implementing Paging
# Simplified example of a paging system
page_table = {}
def load_program(pages):
for logical_page, data in enumerate(pages):
physical_frame = find_free_frame()
page_table[logical_page] = physical_frame
memory[physical_frame] = data
def access_page(logical_page):
physical_frame = page_table.get(logical_page)
if physical_frame is not None:
return memory[physical_frame]
else:
raise Exception("Page Fault!")
def find_free_frame():
# Logic to find a free memory frame
pass
Comparison and Analysis
To better understand the differences between these techniques, consider the following table:
Feature | Contiguous Allocation | Paging | Segmentation |
---|---|---|---|
Memory Division | Single block | Fixed-size pages | Variable segments |
Fragmentation | Both internal & external | Internal only | External |
Ease of Implementation | Simple | Moderate | Complex |
Flexibility | Low | High | High |
Access Speed | Fast | Moderate (due to page table) | Moderate (due to segment table) |
Additional Resources and References
For further reading and an in-depth study of memory management techniques in operating systems, consider the following resources:
- Operating System Concepts by Abraham Silberschatz, Peter B. Galvin, and Greg Gagne.
- Modern Operating Systems by Andrew S. Tanenbaum.
- GeeksforGeeks - Difference between Paging and Segmentation
- TutorialsPoint - Memory Allocation in OS
These resources provide comprehensive insights and practical examples to deepen your understanding of memory management in operating systems.