Disk Scheduling Algorithms
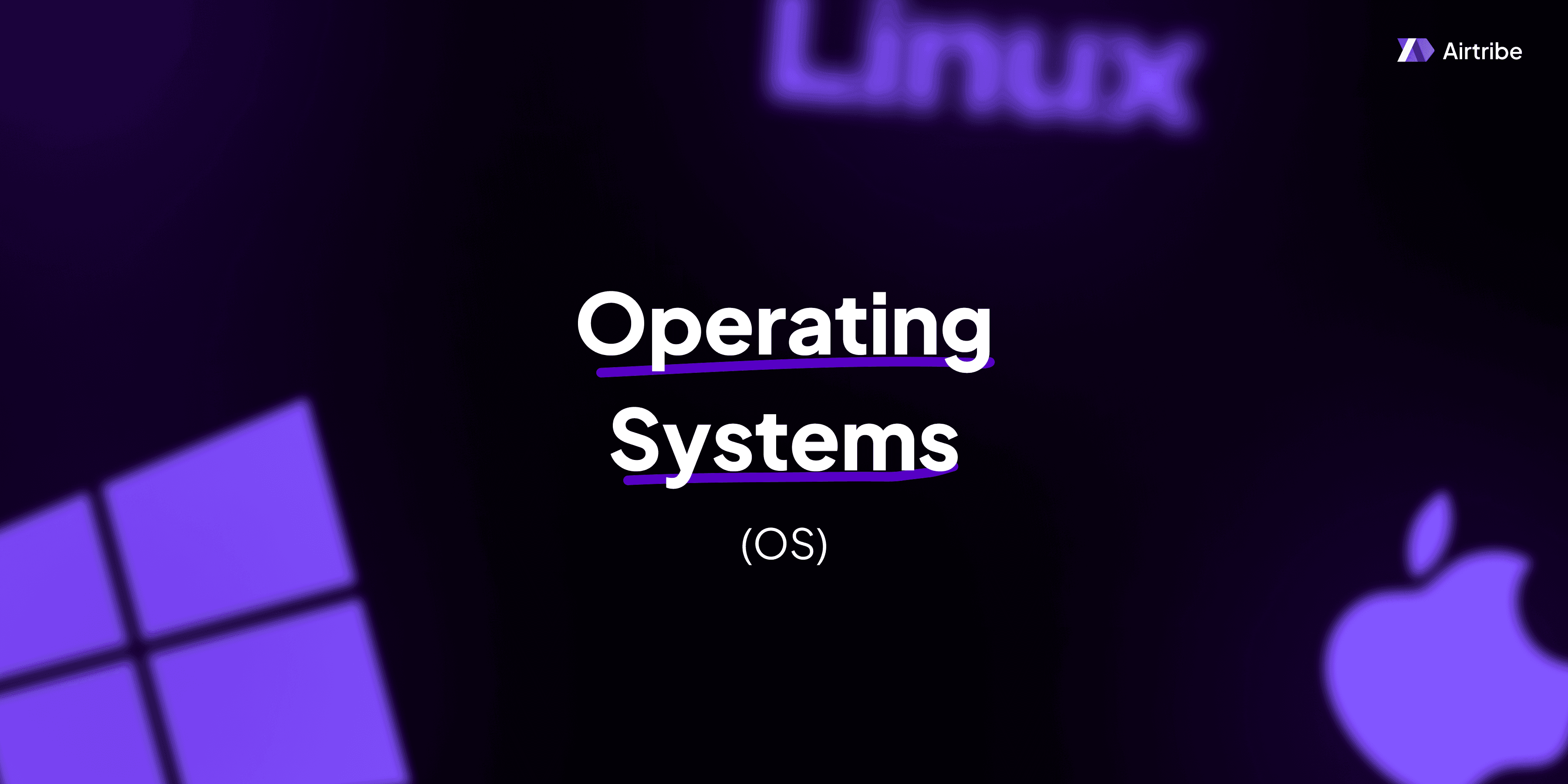
Understanding Disk Scheduling Algorithms
Disk scheduling is a key component of operating systems responsible for effective memory management. It determines the order in which disk I/O requests are processed, enhancing the efficiency of data retrieval processes. Disk scheduling algorithms aim to minimize seek time, improve throughput, and ensure fair usage of the disk by multiple processes. In this article, we will delve into various disk scheduling algorithms, their core concepts, practical applications, and compare their performance.
Core Concepts and Theory
Importance of Disk Scheduling
The primary function of disk scheduling algorithms is to manage the sequence of I/O requests in such a way that non-preemptive disk processes are completed efficiently. This involves reducing the seek time (the time taken for the disk arm to move to the desired track) and maximizing disk performance. With the evolution of multi-user environments and larger datasets, effective disk scheduling is crucial for smoother operations and quick data access.
Key Disk Scheduling Algorithms
First-Come, First-Served (FCFS):
- Functionality: Processes requests in the order they arrive.
- Pros: Easy to implement and understand.
- Cons: Poor performance with heavy traffic, as the arm may move back and forth frequently, causing high average seek time (Convoy Effect).
Shortest Seek Time First (SSTF):
- Functionality: Selects the request closest to the current head position.
- Pros: Reduces total seek time compared to FCFS.
- Cons: Can cause starvation; far requests may never be serviced if closer requests keep coming.
Elevator Algorithm (SCAN):
- Functionality: Moves the arm in one direction first, fulfilling all requests until the end, then reverses direction.
- Pros: Balances seek time better than SSTF and FCFS; prevents starvation.
- Cons: More complex than FCFS, may still head to the end even if few requests are pending.
Circular SCAN (C-SCAN):
- Functionality: Similar to SCAN, except when it reaches one end, it jumps to the start without servicing requests while returning.
- Pros: Provides uniform wait time as every request is treated equally over spans.
- Cons: Increased travel with empty reverse trips.
LOOK and C-LOOK:
- Functionality: Optimizations of SCAN and C-SCAN, where the arm reverses direction or jumps to start only for last requests instead of reaching the very end.
- Pros: Reduce unnecessary travel compared to their SCAN counterparts.
Practical Applications
Real-world Utilization
Disk scheduling is crucial in environments with high disk usage, such as:
- Cloud storage systems, where large numbers of I/O operations need to be managed efficiently.
- Database management systems, requiring quick retrieval and updating of data records.
- Multimedia servers, where sequential access is necessary for streaming activities.
Example Scenario
Consider a situation with I/O request sequences: 98, 183, 37, 122, 14, 124, 65, 67, and the initial position of the head at 53. Different algorithms will approach this sequence uniquely, resulting in different seek times and efficiencies.
Code Implementation and Demonstration
Here's a simple Python script demonstrating the implementation of SSTF:
def calculate_seek_time(head, requests):
seek_sequence = []
completed = [False] * len(requests)
total_seek_time = 0
n = len(requests)
for _ in range(n):
closest = -1
min_distance = float('inf')
for i in range(n):
if not completed[i] and abs(requests[i] - head) < min_distance:
closest = i
min_distance = abs(requests[i] - head)
if closest != -1:
completed[closest] = True
seek_sequence.append(requests[closest])
total_seek_time += abs(requests[closest] - head)
head = requests[closest]
return seek_sequence, total_seek_time
head = 53
requests = [98, 183, 37, 122, 14, 124, 65, 67]
seek_sequence, total_seek_time = calculate_seek_time(head, requests)
print("Seek Sequence:", seek_sequence)
print("Total Seek Time:", total_seek_time)
Comparison and Analysis
To better understand the varied performance of these algorithms, let's compare them based on typical performance metrics:
Algorithm | Average Seek Time | Complexity | Starvation Handling |
---|---|---|---|
FCFS | Generally high | O(n) | Poor |
SSTF | Lower than FCFS | O(n * m) | Risk of starvation |
SCAN | Moderate | O(n) | Fairly good |
C-SCAN | Moderate to high | O(n) | Better than SCAN |
LOOK/C-LOOK | Better than SCAN | O(n) | Fair |
Additional Resources and References
- Books: "Operating System Concepts" by Abraham Silberschatz and "Modern Operating Systems" by Andrew S. Tanenbaum for deeper insights.
- Research Papers: Explore journals and papers on optimizations in disk scheduling for advanced understanding.
- Online Courses: Platforms like Coursera and Udacity offer courses on operating systems that cover disk scheduling in detail.
Disk scheduling algorithms play a pivotal role in efficient memory management within operating systems, directly impacting the overall system performance. Understanding these algorithms equips software engineers with the knowledge to design and optimize systems for better resource management and operation efficiency.