file allocation and deallocation
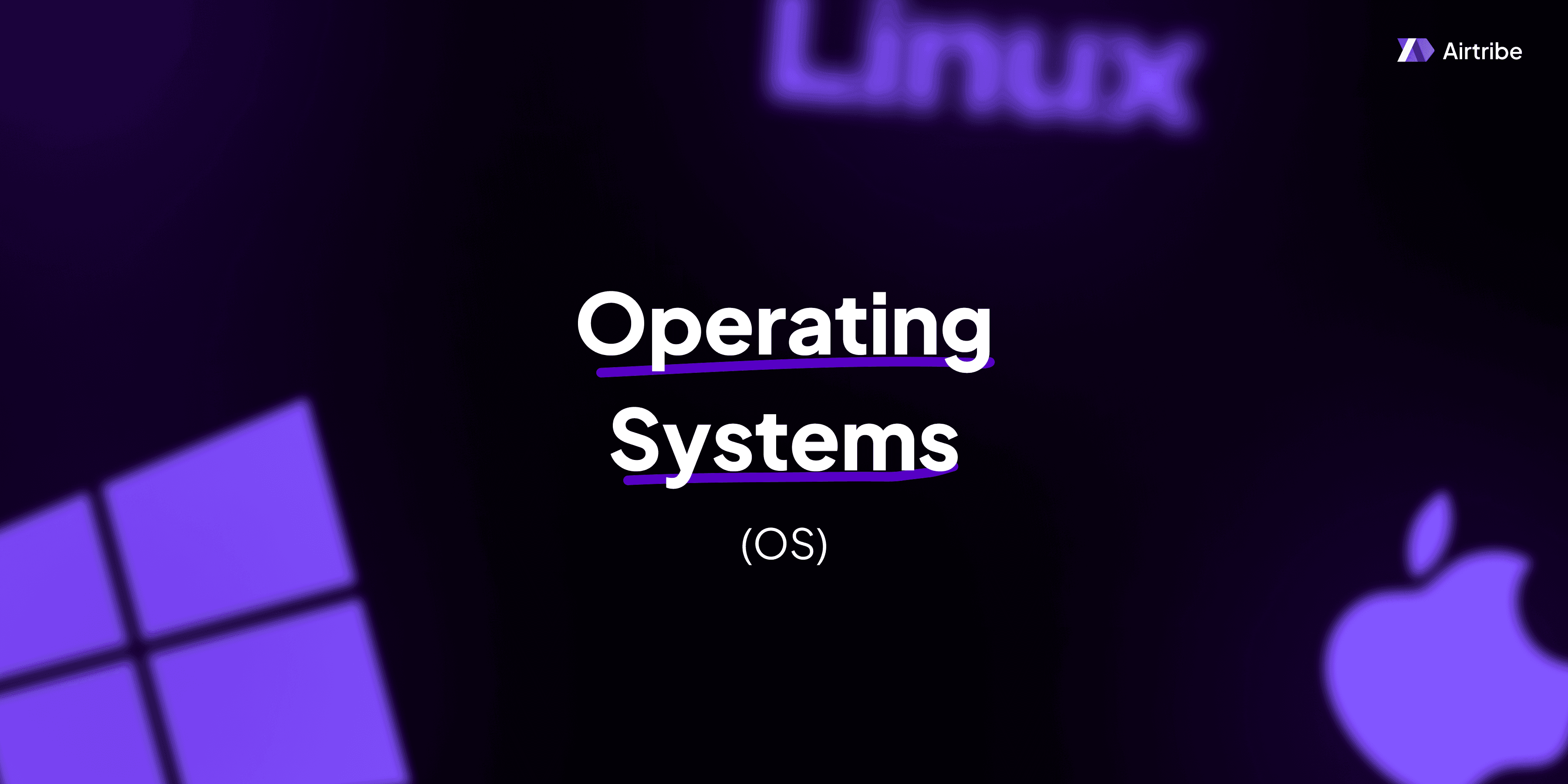
Understanding File Allocation and Deallocation
Efficient file management in computer systems is crucial for optimizing resource usage and ensuring data integrity. File allocation and deallocation are core functionalities within operating systems' file systems, governing how files are stored and removed from disk storage. This article provides a comprehensive overview of these processes within file systems, exploring core concepts, methodologies, and implications.
Core Concepts and Theory
File Allocation
File allocation is the method through which an operating system assigns disk space to files. It determines the data structure used to store and organize files on storage media. The following are common file allocation methods:
Contiguous Allocation:
- Concept: Stores files in contiguous blocks of disk space.
- Advantages: Simple to implement and offers fast access due to minimal seeking.
- Disadvantages: Prone to fragmentation, which may lead to inefficient storage utilization over time.
Linked Allocation:
- Concept: Files are stored in linked blocks. Each block contains a pointer to the next block in the file sequence.
- Advantages: Overcomes fragmentation issues inherent in contiguous allocation.
- Disadvantages: Can result in slower access times due to the need to follow pointers.
Indexed Allocation:
- Concept: Uses an index block with pointers to all other blocks occupied by the file.
- Advantages: Easy access to any file block and can handle random access well.
- Disadvantages: Requires additional space for the index, which can be a limitation for small files.
Multilevel Index:
- Concept: Combines aspects of the indexed allocation but allows for multiple layers of indices, thus supporting larger files.
- Advantages: Efficient for handling very large files.
- Disadvantages: Increasing layers of indices can complicate the file system's structure.
File Deallocation
File deallocation refers to the process of releasing space occupied by files when they are deleted. Proper deallocation is essential to prevent resource leaks and ensure that space is available for future allocations. Key methods include:
- Immediate Deallocation: The space is released immediately when the file is deleted.
- Deferred Deallocation: Space is marked as free but not immediately available until system conditions are optimal to carry out the deallocation without impacting performance.
File deallocation must also address issues such as ensuring data consistency and updating metadata to reflect changes.
Practical Applications
File allocation and deallocation methods significantly impact systems where performance and efficiency are critical, such as:
- Database Systems: Efficient storage access patterns are essential for high-performance queries.
- Media Streaming Services: Rapid file access minimizes buffer lag.
- Operating System Boot Time: Quick loading times often rely on effective file organization and access.
Code Implementation and Demonstrations
Below is a simple pseudocode implementation of Linked Allocation demonstrating file allocation:
class Block:
data = null
nextBlockPtr = null
class File:
startBlockPtr = null
def allocate(file, dataBlocks):
currentBlock = file.startBlockPtr
for data in dataBlocks:
newBlock = Block()
newBlock.data = data
if currentBlock != null:
currentBlock.nextBlockPtr = newBlock
currentBlock = newBlock
def deallocate(file):
currentBlock = file.startBlockPtr
while currentBlock != null:
temp = currentBlock
currentBlock = currentBlock.nextBlockPtr
delete temp
This pseudocode demonstrates linked allocation where files are stored across discrete blocks linked via pointers. The deallocate function frees the memory used by each block.
Additional Resources and References
For a deeper understanding of file allocation and deallocation practices, the following resources are recommended:
- "Operating System Concepts" by Abraham Silberschatz et al.: Provides a thorough grounding in operating system principles, including file system details.
- "Modern Operating Systems" by Andrew S. Tanenbaum: Offers insights into the design and implementation of modern operating systems with a focus on file system management.
Familiarizing oneself with these foundational texts can enhance one's comprehension of various allocation strategies' practical impacts and trade-offs.