Process synchronization and its tools
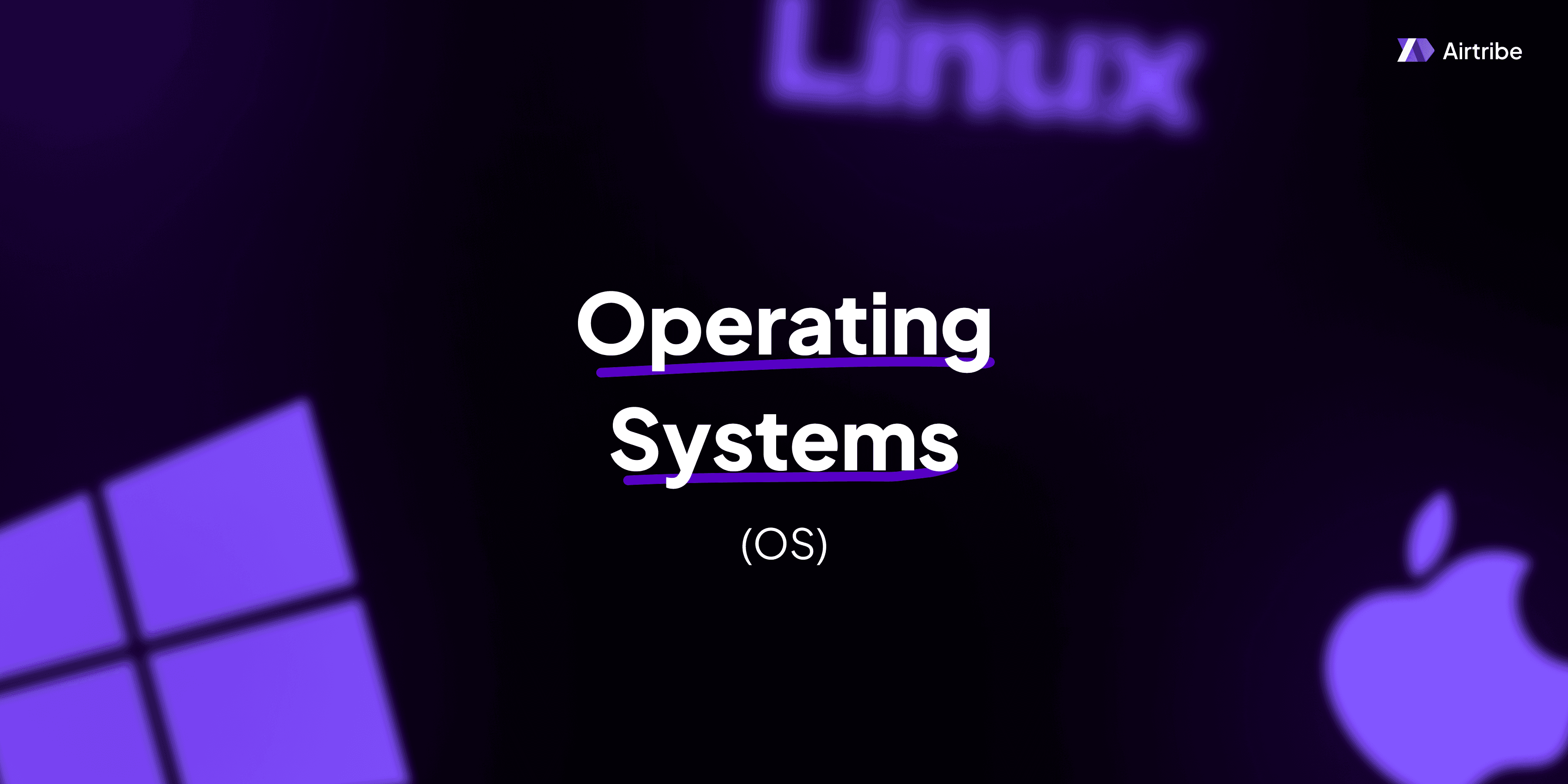
Understanding Process Synchronization in Operating Systems
Process synchronization is a vital concept in operating systems that deals with the coordination of processes to ensure that they execute in a predictable and orderly fashion. It becomes particularly important in a multitasking and multi-user environment, where several processes may need to access shared resources simultaneously. Without proper synchronization, these concurrent processes can lead to inconsistencies and corrupt data.
Core Concepts and Theory
Process synchronization primarily revolves around ensuring that processes do not interfere with each other while accessing shared resources or data. Here are some of the fundamental concepts and theories:
Critical Section Problem
The critical section is a part of the code where shared resources are accessed. The critical section problem ensures that when one process is executing in its critical section, no other process is allowed to execute in its critical section.
Race Condition
A race condition occurs when the system's behavior depends on the relative timing of events, such as threads or processes accessing shared data concurrently. This can lead to inconsistent results.
Mutual Exclusion
Mutual exclusion is essential to prevent multiple processes from entering their critical sections simultaneously. Only one process should be allowed in the critical section to ensure consistency.
Progress
If no process is executing in the critical section, and some processes wish to enter their critical sections, then selection of processes that will enter the critical section next cannot be postponed indefinitely (i.e., there is progress).
Bounded Waiting
A solution to the critical section problem must impose a bound on the number of times that other processes are allowed to enter their critical sections after a process has requested to enter its critical section and before that request is granted.
Tools for Process Synchronization
Several tools and mechanisms are used to achieve process synchronization efficiently:
Semaphores
Semaphores are synchronization primitives that use counters to manage concurrent processes' access to shared resources. They are of two types:
- Binary Semaphore (mutex): Acts as a lock. It can take only two values: 0 or 1. It's used for simple locking mechanisms.
- Counting Semaphore: Used to control access to a resource pool consisting of a finite number of instances.
Monitors
Monitors are high-level synchronization constructs that provide a mechanism for threads to safely access shared data. A monitor uses condition variables and locks to control thread access to shared resources.
Locks
Locks are used to enforce limits on concurrent access to a resource. They ensure that only one process can access the shared resource or critical section at any time.
Conditional Variables
Conditional variables allow threads to wait for certain conditions to be true before continuing execution. They help in managing the synchronization of threads.
Barriers
Barriers are synchronization methods used to block a set of threads until all threads reach a certain point. They are useful in parallel computing where tasks need to be synchronized at certain execution points.
Practical Applications
In the realm of operating systems, process synchronization is crucial in various scenarios, such as:
Producer-Consumer Problem: Here, synchronization ensures that producers do not produce data when the buffer is full and consumers do not consume data when the buffer is empty.
Dining Philosophers Problem: This is a classic synchronization problem dealing with resource sharing between multiple processes. It emphasizes resource allocation and synchronization.
Readers-Writers Problem: This problem highlights synchronization where multiple readers can read simultaneously but only one writer can write at the same time.
Code Implementation and Demonstrations
To illustrate synchronization, let's look at a simple implementation of a binary semaphore (mutex) for critical section management in Python using threading:
import threading
# Shared resource
counter = 0
# Creating a lock
lock = threading.Lock()
def increment():
global counter
with lock: # Acquiring the lock
temp = counter
temp += 1
counter = temp
threads = []
for i in range(100):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f'Final counter value: {counter}')
In this example, a lock is used to synchronize the increment
function, ensuring the critical section that updates counter
is accessed by only one thread at a time.
Additional Resources and References
Books:
- "Operating System Concepts" by Abraham Silberschatz, Peter B. Galvin, and Greg Gagne.
- "Modern Operating Systems" by Andrew S. Tanenbaum and Herbert Bos.
Online Courses:
- Operating Systems and You: Becoming a Power User (Coursera)
- Operating Systems SDE (Khan Academy)
Websites:
- GeeksforGeeks: Operating System Synchronization
- TutorialsPoint: OS Process Synchronization
Process synchronization forms the backbone of efficient and safe process management within operating systems. By employing various synchronization tools such as semaphores, monitors and locks, it ensures that processes operate without conflict and accurately share resources, maintaining data integrity and system reliability.