Producer-consumer problem
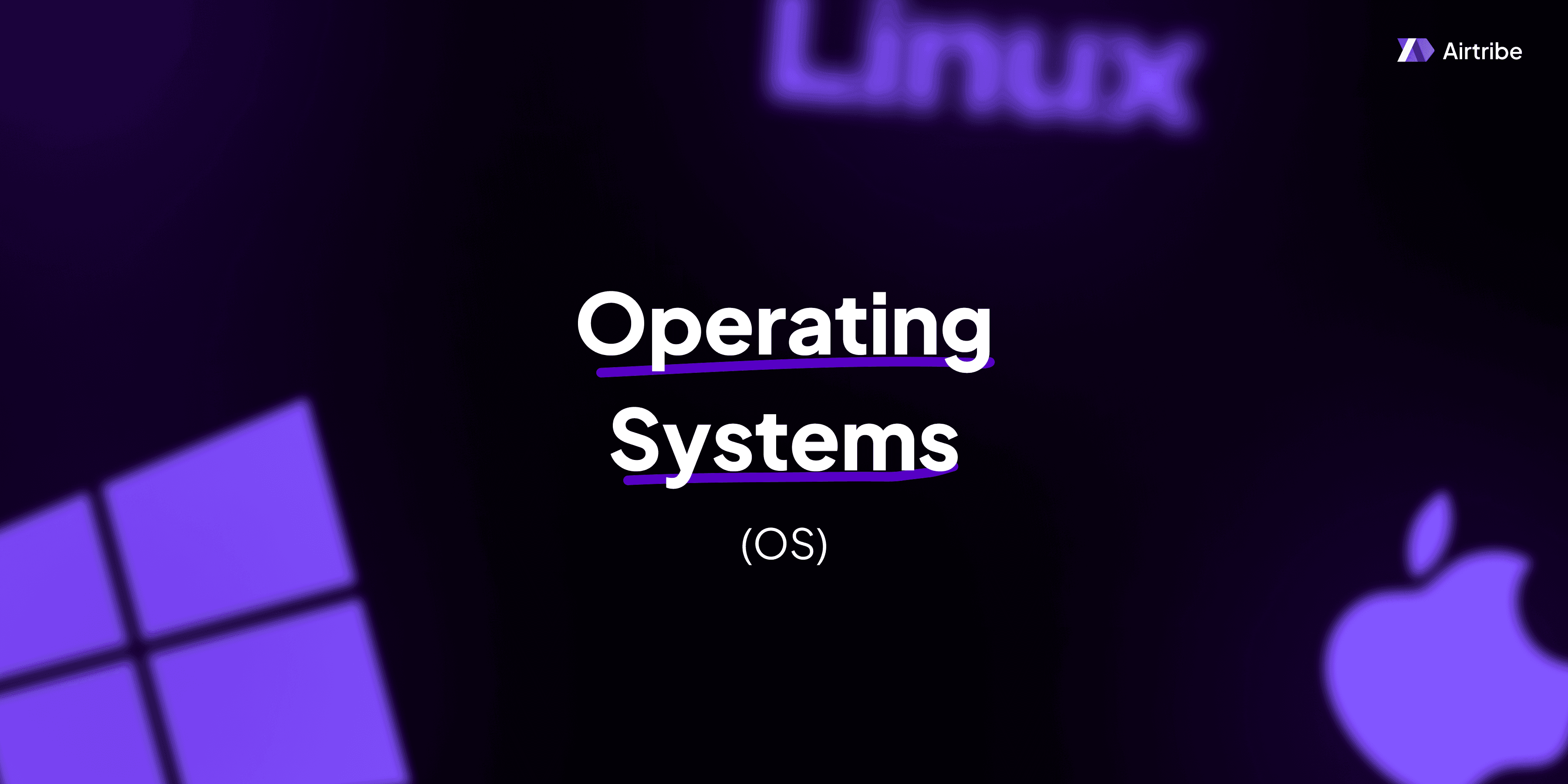
Understanding the Producer-Consumer Problem
The Producer-Consumer problem is a classic example of a multi-process synchronization issue in operating systems. It highlights the challenges of managing shared resources among concurrent processes, making it a fundamental concept for process management and synchronization.
Core Concepts and Theory
In the Producer-Consumer problem, two types of processes, producers and consumers, share a common buffer. The producers are responsible for adding items to the buffer, while the consumers remove items. The primary challenge is coordinating these processes to avoid scenarios like buffer overflow (when producers try to add items to a full buffer) or buffer underflow (when consumers try to remove items from an empty buffer).
Key Concepts:
- Buffer: A finite-sized, shared space where the producer can add items and the consumer can remove items.
- Synchronization: Mechanism to ensure that the buffer is not accessed simultaneously by multiple processes, preventing race conditions.
- Mutex Locks: Used to protect shared resources by ensuring that only one process can access the buffer at any time.
- Semaphore: A signaling mechanism used to synchronize operations by controlling access to shared resources with counter variables.
Practical Applications
The Producer-Consumer problem models various real-world applications such as:
- Print Spoolers: Where documents are queued by producers and printed by consumers.
- Multithreaded Applications: Where data is produced at one rate and consumed at another, necessitating a synchronized exchange.
- Data Streaming: Such as video buffering, where data packets are produced and consumed.
Code Implementation and Demonstrations
The Producer-Consumer problem can be implemented using different synchronization tools. Here, we'll demonstrate a solution using semaphores and mutex locks in a pseudo-code format.
# Initializing buffer and synchronization primitives
buffer = Queue(maxsize=5)
mutex = threading.Lock()
empty = threading.Semaphore(5) # Buffer empty slots
full = threading.Semaphore(0) # Buffer filled slots
def producer():
while True:
item = produce_item()
empty.acquire()
mutex.acquire()
buffer.put(item)
mutex.release()
full.release()
def consumer():
while True:
full.acquire()
mutex.acquire()
item = buffer.get()
mutex.release()
empty.release()
consume_item(item)
Explanation:
- Mutex and Semaphores: Here,
mutex
ensures mutual exclusion while accessing the buffer, whileempty
andfull
are counting semaphores tracking buffer availability. - Process Flow: The producer creates an item and waits for an empty slot using
empty.acquire()
before entering the critical section. The consumer waits for an item usingfull.acquire()
and then processes it.
Comparison and Analysis
Different Approaches:
- Blocking Approach: As shown in the above example, threads block when the buffer is full/empty until conditions change.
- Non-blocking Approach: Uses techniques like busy-waiting, where processes continuously check buffer status without blocking, leading to resource wastage.
- Bounded Buffer Solution: Ensures a fixed-size buffer, preventing operations from proceeding when conditions aren't favorable.
Analysis
- Efficiency: The semaphore/mutex approach is efficient and widely used due to its non-busy-waiting nature.
- Complexity: The non-blocking approach might be less efficient in terms of CPU usage.
- Applicability: Choosing between techniques depends on application needs, like time sensitivity versus resource conservation.
Additional Resources and References
To deepen understanding of the Producer-Consumer problem, explore the following resources:
- Books: "Operating System Concepts" by Abraham Silberschatz for comprehensive OS synchronization principles.
- Online Tutorials: Websites like GeeksforGeeks and TutorialsPoint offer detailed article series on synchronization problems.
- Research Papers: Explore IEEE journals for advanced solutions and variants of the Producer-Consumer problem applied in modern computing scenarios.
By mastering the Producer-Consumer problem, software development engineers gain crucial insights into process synchronization, enabling them to design more reliable and efficient multi-process systems.