Thrashing
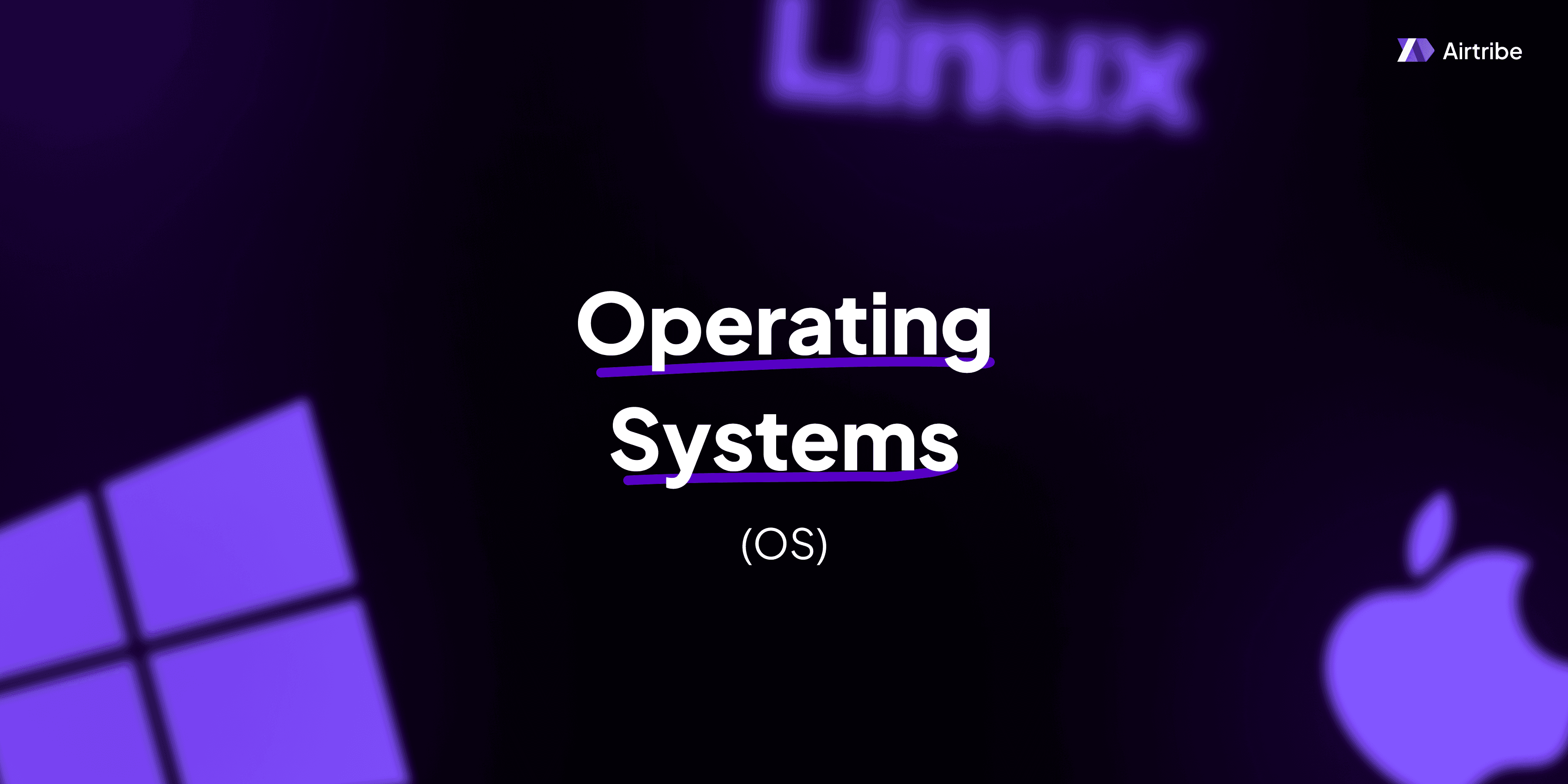
Understanding Thrashing in Operating Systems
In the realm of computer science, particularly in operating systems, "thrashing" is a critical performance issue related to memory management. When an operating system spends more time swapping pages in and out of memory rather than executing instructions, the system is experiencing thrashing. This article explores the concept of thrashing, the core theory behind it, and strategies to mitigate its effects.
Core Concepts and Theory
What is Thrashing?
Thrashing occurs when a computer's virtual memory subsystem is in a constant state of high paging activities. The CPU spends most of its time swapping pages instead of executing instructions, leading to severe system slowdown or even complete system freeze.
The phenomenon is often triggered when the system's working set of processes does not fit into physical memory, causing an excessive number of page faults. A page fault occurs when a program tries to access a page not currently mapped to the physical memory, prompting the operating system to retrieve it from secondary storage (disk).
Working Set Model
The working set model plays a pivotal role in understanding thrashing. The working set is defined as the set of pages actively used by a process during a specific time interval. If the working set fits into memory, the process will run efficiently with minimal page faults. However, as the total working set size exceeds available memory, thrashing can occur.
Page Fault Frequency
The frequency of page faults is an indicator of thrashing. In a non-thrashing state, the system observes a stable and low page fault rate. When thrashing begins, this rate sharply increases due to processes frequently needing pages not present in memory.
Practical Applications
Mitigating Thrashing
Operating systems employ several strategies to control and minimize thrashing:
Increase Physical Memory: Adding more RAM can provide a larger buffer for the working set, effectively reducing thrashing.
Adjust Multiprogramming Level: Limiting the number of concurrent processes can help reduce memory contention. Only executing a set number of processes limits the working set size.
Page Replacement Algorithms: Utilizing efficient page replacement strategies, such as Least Recently Used (LRU) or Most Recently Used (MRU), can help optimize which pages remain in memory, thus reducing page faults.
Locality of Reference: Programming techniques that enhance locality of reference can be employed. Accessing data or instructions in clustered sequences can lower page replacement activity.
Code Implementation and Demonstrations
Thrashing is a systemic issue and not typically tackled with a straightforward code solution. However, understanding and simulating it can be valuable for educational purposes. Consider the following pseudocode which simulates a simplistic situation leading to thrashing:
import random
def simulate_thrashing(virtual_pages, physical_frames, operations):
memory = [-1] * physical_frames
page_faults = 0
for operation in range(operations):
page = random.choice(virtual_pages)
if page not in memory:
page_faults += 1
replace_index = random.randint(0, physical_frames - 1)
memory[replace_index] = page
print(f"Operation {operation}: Page {page}, Memory State: {memory}")
print(f"Total Page Faults: {page_faults}")
simulate_thrashing(virtual_pages=[1, 2, 3, 4, 5], physical_frames=3, operations=20)
This simple simulation represents random page accesses and replacements, leading to frequent page faults when the number of physical frames is insufficient.
Additional Resources and References
For a comprehensive understanding of thrashing and memory management patterns, the following resources may prove beneficial:
- Operating Systems: Internal and Design Principles by William Stallings
- Modern Operating Systems by Andrew S. Tanenbaum
- Research papers on memory management techniques available in ACM and IEEE journals
Understanding and mitigating thrashing are crucial aspects of designing efficient operating systems. Through informed memory management techniques, the challenge of thrashing can be effectively handled, ensuring optimal system performance.