Pull vs. Push
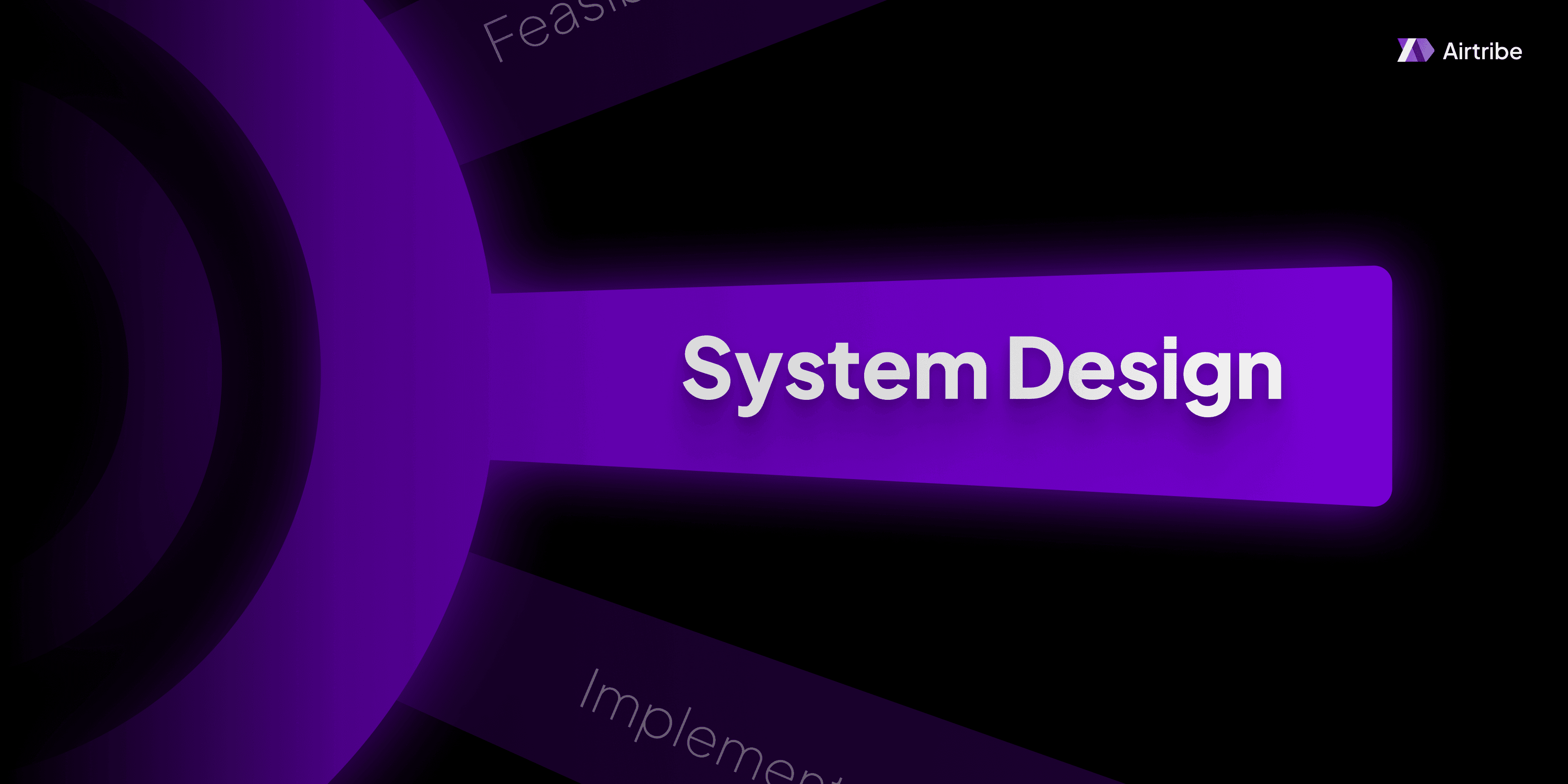
Understanding Pull vs. Push in System Design
Designing a robust and efficient system often involves critical decisions regarding data flow and communication between system components. One such fundamental decision is choosing between pull and push models. Each model has its advantages and tradeoffs, which significantly impact system architecture, performance, and scalability. In this article, we explore the core concepts, compare both models, and discuss practical applications to help guide your system design.
Core Concepts and Theory
Pull Model
The pull model is based on the passivity of data sources, where data consumers are responsible for requesting (or "pulling") the needed data. This model ensures that consumers retrieve the most current data as needed, reducing unnecessary data transmission.
Characteristics of Pull Model:
- Data Requests: Consumers initiate communication.
- Latency: Affects response times, as data retrieval starts only on request.
- Bandwidth Efficiency: Data is transferred only when requested. This can reduce unnecessary data flow.
- Complexity: Consumers must implement logic for data retrieval, potentially increasing complexity.
Push Model
Conversely, the push model involves proactive data sources, where updates are sent ("pushed") to consumers as soon as changes occur. This approach keeps consumers updated in real time but may result in redundant data transfer if updates occur frequently.
Characteristics of Push Model:
- Data Delivery: Sources initiate communication.
- Latency: Offers low latency since updates are automatically sent to consumers.
- Bandwidth Usage: Higher potential for bandwidth overuse due to continuous data streaming.
- Complexity: Requires robust infrastructure to handle frequent updates and potential data overload.
Practical Applications
Choosing the Right Model
The decision between pull and push models should align with the specific requirements of the application:
Real-time Systems: Push model is ideal, providing immediate updates as required in gaming, live streaming, or stock trading applications.
Resource-Constrained Environments: Pull model can conserve bandwidth and minimize resource usage, suitable for mobile apps or environments with intermittent network connectivity.
Hybrid Approaches
In practice, systems often implement a combination of both models to optimize performance:
- Polling: A variant of the pull model where consumers periodically check for updates, balancing between timely updates and bandwidth usage.
- WebHooks: A push model strategy where data is pushed to consumers upon specific events, commonly used in web development.
Code Implementation and Demonstrations
To illustrate both models, let's consider a simple example in a web application context with REST APIs (for pull) and WebSocket (for push).
Pull Model with REST API:
import requests
def get_data():
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
return response.json()
else:
raise Exception("Failed to retrieve data")
# Usage
data = get_data()
print(data)
Push Model with WebSocket:
const WebSocket = require('ws');
const ws = new WebSocket('wss://example.com/socket');
ws.on('open', function open() {
console.log('Socket connected');
});
ws.on('message', function incoming(data) {
console.log('Received data:', data);
});
Comparison and Analysis
Aspect | Pull Model | Push Model |
---|---|---|
Initiative | Client (Consumer) | Server (Producer) |
Latency | Higher | Lower |
Bandwidth Usage | More efficient | Potential overuse |
Complexity | Consumer-side complexity | Producer-side complexity |
Real-time Suitability | Moderate | High |
Scalability Considerations:
- Pull Model: Scales well in environments with diverse consumer capabilities and is less likely to overwhelm network bandwidth.
- Push Model: Requires infrastructure capable of handling high concurrency and large-scale data dissemination.
Additional Resources and References
Books:
- Designing Data-Intensive Applications by Martin Kleppmann
- Building Microservices by Sam Newman
Online Articles:
- "REST vs WebSockets: Understanding Their Differences" - Smashing Magazine
- "System Design Basics" - Educative.io
Web Tutorials:
- Codecademy: Overview of APIs
- WebSockets: How do they differ from HTTP?
Understanding the nuances between pull and push in system design helps create efficient, scalable systems aligned with your application's specific needs. By carefully evaluating tradeoffs, you can choose the appropriate model to facilitate seamless data communication.