What is a Single Point of Failure?
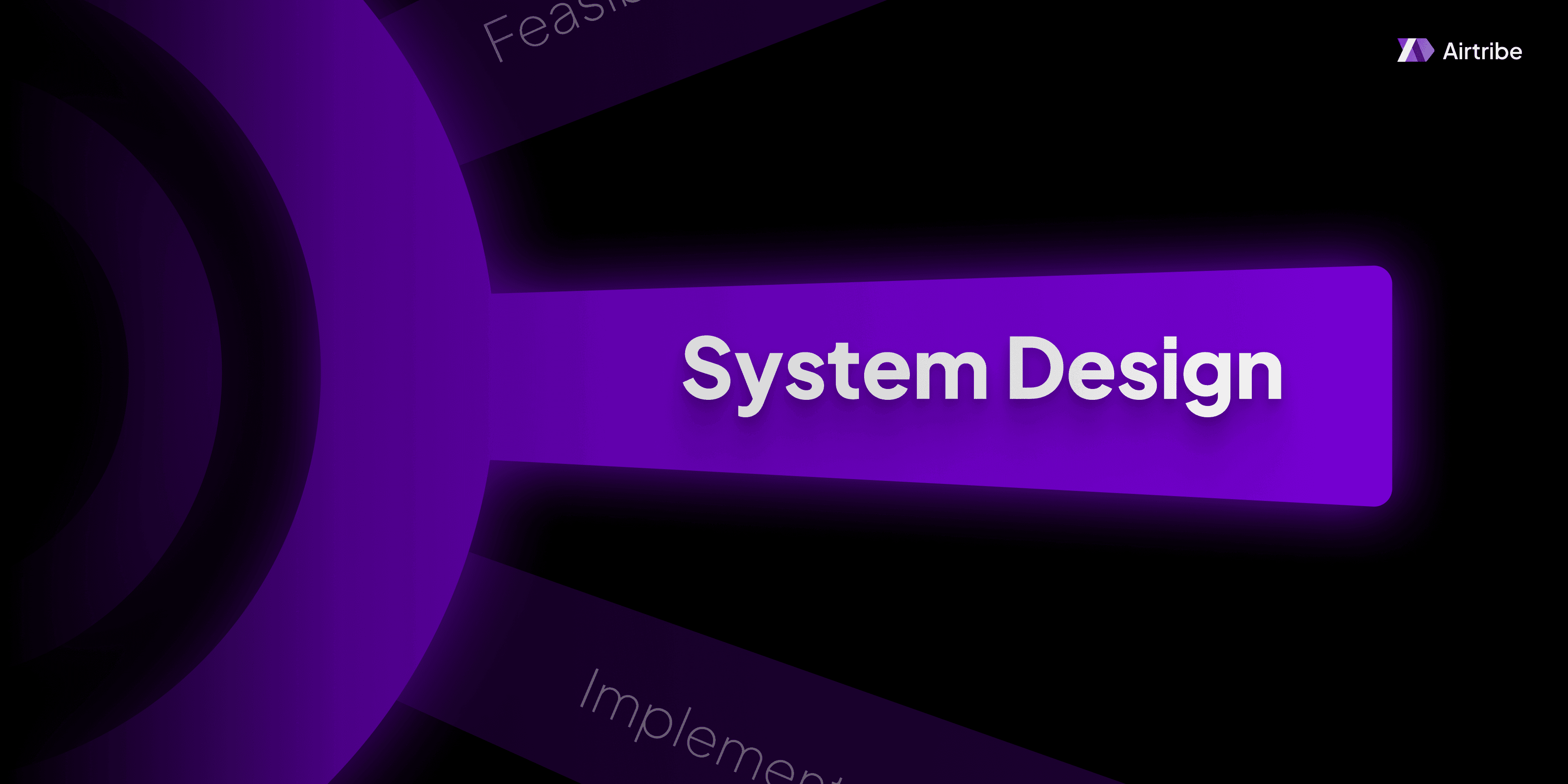
Understanding Single Point of Failure in System Design
In the realm of DevOps and system design, the term "Single Point of Failure" (SPOF) refers to a component within a system that, if it were to fail, would lead to the entire system's collapse or significant degradation in performance. It's a critical concept that engineers must address to design robust, reliable, and fault-tolerant systems.
Core Concepts and Theory
A Single Point of Failure can exist in various forms, including hardware, software, or human processes. When a system heavily relies on a single component, the failure of that component can result in downtime, data loss, or service disruption. Understanding and mitigating SPOFs is pivotal to enhancing a system's resilience.
Characteristics of SPOF
- Centralization: If a component plays a central role in system operations without backup, it is classified as an SPOF.
- Criticality: The importance of the component in maintaining system functionality.
- Lack of Redundancy: Absence of backup components to take over in case of failure.
Identifying Single Points of Failure
To identify SPOFs, system architects should conduct thorough analyses, considering both hardware and software components:
- Dependency Analysis: Examine system architecture to identify dependencies. Components that numerous processes rely on are potential SPOFs.
- Failure Mode Analysis: Determine how each component might fail and the potential impact on the whole system.
- Historical Failure Data: Use past incidents and failure data to identify weak links in the system structure.
Practical Applications
In practice, addressing SPOFs involves strategies that enhance redundancy and reduce dependency risks:
- Redundancy Implementation: Employ multiple instances of critical components. For example, in cloud services, load balancers distribute traffic across multiple servers.
- Failover Mechanisms: Automatically switch operations to a standby system or network upon component failure.
- Decentralizing Critical Functions: Disperse vital system functions over multiple components or services to reduce impact.
Code Implementation and Demonstrations
While exact code configurations depend on the specific technology stack and system architecture, here's a generic Python example demonstrating a simple failover mechanism to handle database failover using a primary and secondary database connection:
import psycopg2
def connect_to_database(db_config):
try:
conn = psycopg2.connect(**db_config)
print("Connection successful")
return conn
except psycopg2.OperationalError as e:
print(f"Connection failed: {e}")
return None
primary_db_config = {
'host': 'primary.db.server',
'database': 'appdb',
'user': 'appuser',
'password': 'password123'
}
secondary_db_config = {
'host': 'secondary.db.server',
'database': 'appdb',
'user': 'appuser',
'password': 'password123'
}
# Try connecting to the primary database
conn = connect_to_database(primary_db_config)
# If connecting to the primary fails, try connecting to the secondary
if conn is None:
print("Switching to secondary database...")
conn = connect_to_database(secondary_db_config)
# Proceed with database operations if a connection was successful
if conn:
# Perform database operations
conn.close()
else:
print("All connections failed. Please check configurations.")
Comparison and Analysis
Understanding the behavior and handling of SPOF is crucial. Here is a comparative analysis of systems with and without SPOF measures in place:
Feature | Systems with SPOF | Systems without SPOF |
---|---|---|
Reliability | Low | High |
Resilience | Low | High |
Maintenance Complexity | Simple but risky | Complex but secure |
Cost | Lower hardware costs | Higher due to redundancy |
Downtime Impact | Significant | Minimal |
Additional Resources and References
To gain deeper insights into SPOF and advanced strategies for mitigation, consider exploring the following resources:
- Site Reliability Engineering: How Google Runs Production Systems by Niall Richard Murphy et al.
- The Phoenix Project: A Novel about IT, DevOps, and Helping Your Business Win by Gene Kim, Kevin Behr, and George Spafford
- AWS Well-Architected Framework
- Google Cloud Architecture Framework
By comprehending the significance of Single Points of Failure and employing effective strategies, organizations can enhance system reliability and ensure continuous service availability, which is the cornerstone of robust system design in today’s digital era.